As a full-stack web developer, exploring into the world of Laravel can significantly amplify your capabilities.
In this comprehensive guide, we’ll walk through the essential steps for mastering Laravel—a powerful PHP framework that simplifies full-stack web development.
Quick Links
Why Master Laravel?
Laravel has become a cornerstone in modern web development, renowned for its elegant syntax, robust features, and developer-friendly environment.
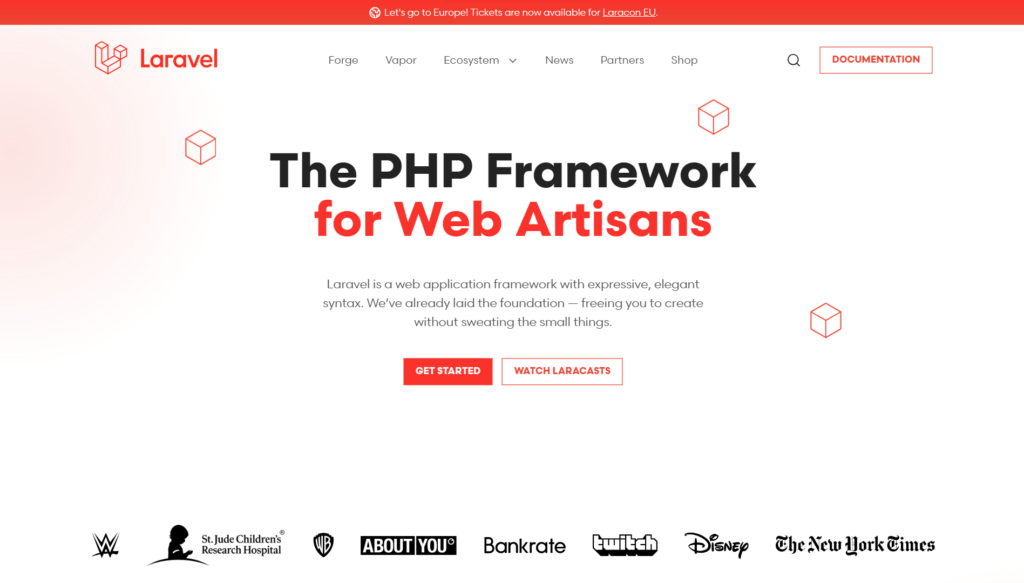
For aspiring full-stack web developers, gaining proficiency in Laravel opens doors to streamlined workflows, efficient coding practices, and the ability to craft scalable, maintainable applications.
Throughout this guide, we’ll break down the learning process into digestible sections, ensuring a smooth transition from novice to expert. Let’s embark on this journey to empower your full-stack aspirations.
Understanding the Basics
Laravel is a PHP web application framework designed for elegant syntax and ease of use.
Make sure you have already installed Composer. If you have not installed Composer in your computer, you can download at https://getcomposer.org/download/.
To get started, you’ll need to install Laravel.
Open your terminal and run:
composer create-project --prefer-dist laravel/laravel your-project-name
This command fetches the latest Laravel version and sets up a new project for you.
Installation and Setup Guide for Beginners
Once installed, Laravel offers a structured and intuitive environment. Familiarize yourself with the directory structure and key files, such as routes/web.php
where you define your web routes.
Route::get('/', function () {
return view('welcome');
});
Overview of MVC Architecture
Laravel follows the Model-View-Controller (MVC) architecture. Models interact with the database, views represent the user interface, and controllers manage the application logic.
Understanding this architecture lays the foundation for building scalable and organized applications.
In the next section, we’ll explore into building a strong foundation by exploring it’s Blade templating engine.
Building a Strong Foundation
Mastering these foundational elements sets the stage for a smoother learning journey.
Exploring Blade Templating Engine
The Laravel’s Blade templating engine simplifies the creation of views with its intuitive syntax.
Let’s create a basic Blade view:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Welcome to Blade</title>
</head>
<body>
<h1>Hello, {{ $name }}!</h1>
</body>
</html>
Here, {{ $name }}
is a Blade directive that outputs the value of the $name
variable. Blade makes it easy to integrate dynamic content into your views.
Understanding Eloquent ORM for Database Interaction
Eloquent ORM simplifies database interactions in Laravel. Define models and eloquently query the database.
For instance, let’s create a simple Task
model:
php artisan make:model Task
Now, in Task.php
, define the model:
class Task extends Model
{
// Model logic here
}
Now, you can query tasks from the database effortlessly:
$tasks = Task::all();
Introduction to Artisan Commands
The Artisan commands streamline various development tasks. For example, create a controller with:
php artisan make:controller MyController
This command generates a controller file, allowing you to focus on writing logic rather than boilerplate code.
Basic Features
Mastering these features equips you with the tools to create dynamic and secure web applications.
In-depth Exploration of Routing
Laravel’s routing system simplifies defining application routes. In your routes/web.php
file, create routes like this:
Route::get('/about', function () {
return 'Welcome to the About page!';
});
Route::post('/submit', 'FormController@submitForm');
This example demonstrates a basic GET route and a POST route. Understanding routing is pivotal for defining your application’s navigation.
Utilizing Middleware for Enhanced Application Security
Middleware in Laravel provides a convenient mechanism to filter HTTP requests.
For instance, implement authentication middleware to secure routes:
Route::get('/dashboard', 'DashboardController@index')->middleware('auth');
This ensures that only authenticated users can access the dashboard. Leveraging middleware enhances your application’s security and control.
Leveraging Built-in Authentication System
Laravel comes with a robust authentication system out of the box. Use Artisan to scaffold the necessary controllers and views:
php artisan make:auth
This command sets up registration, login, and password reset functionality, allowing you to focus on building unique features for your application.
Working with Frontend Technologies
Implementing frontend best practices ensures a seamless user experience across different devices.
Popular Frontend Framework Integration
Laravel seamlessly integrates with frontend frameworks like Vue.js and React, enhancing the interactivity of your applications. Let’s take Vue.js as an example:
- Install Vue.js:
npm install vue
- Create a Vue component in your
resources/js/components
directory. - Include the component in your Blade view:
<div id="app">
<example-component></example-component>
</div>
<script src="{{ mix('js/app.js') }}"></script>
This integration allows you to build dynamic, reactive interfaces while leveraging its backend capabilities.
Using Laravel Mix for Asset Compilation and Optimization
Laravel Mix simplifies asset compilation and optimization. Define your asset compilation in webpack.mix.js
:
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css');
Then, run the compilation with:
npm run dev
Laravel Mix streamlines the process, making it easier to manage your application’s assets.
Best Practices for Crafting Responsive Interfaces
Ensure your applications are user-friendly and responsive. Use responsive design principles and libraries like Bootstrap to create interfaces that adapt to various screen sizes:
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
Advanced Topics
As you explore into these advanced topics, you’ll gain a deeper understanding of its capabilities.
Introduction to API Development
Laravel simplifies API development, allowing your applications to communicate with external services or mobile apps.
Create an API endpoint with:
Route::get('/api/data', 'ApiController@getData');
In the corresponding controller, return data as JSON:
public function getData()
{
$data = // retrieve data logic
return response()->json($data);
}
Understanding API development extends the reach and functionality of your web applications.
Exploring Laravel Packages and Extending Functionality
Laravel’s extensive ecosystem includes packages that enhance functionality. Use Composer to install packages:
composer require vendor/package-name
For example, the laravel/ui
package adds frontend scaffolding:
composer require laravel/ui
php artisan ui bootstrap --auth
Explore packages to find solutions that accelerate your development process.
Tips for Optimizing Your Web Applications for Performance
Optimizing your web application is crucial for performance. Utilize caching mechanisms, database indexing, and eager loading to reduce response times.
Additionally, configure Laravel’s task scheduler for background tasks:
// In App\Console\Kernel.php
protected function schedule(Schedule $schedule)
{
$schedule->command('inspire')->hourly();
}
These optimizations ensure your application runs smoothly, even as it scales.
Testing and Debugging
By mastering debugging techniques, you’ll be equipped to identify and resolve issues swiftly, ensuring the stability of your web applications.
Overview of the Testing Tools
Laravel provides a robust testing environment to ensure the reliability of your code. Leverage PHPUnit for unit testing and Laravel Dusk for browser testing.
- Run PHPUnit tests:
php artisan test
- Execute Dusk tests:
php artisan dusk
Writing tests helps identify and rectify issues early in the development process.
Debugging Techniques for Troubleshooting and Optimization
Debugging is a crucial skill for any developer. Laravel’s integrated debugging tools make the process more efficient.
- Utilize the built-in logging:
Log::info('This is an informational message.');
- Leverage breakpoints and step-through debugging with Xdebug for a detailed analysis.
In the upcoming sections, we’ll explore deployment and maintenance strategies, guiding you through the process of taking your web project from development to production.
Deployment and Maintenance
By following these deployment and maintenance practices, you ensure the reliability and security of your web application in a production environment.
Best Practices for Deploying Your Web Applications
Deploying a Laravel application involves several key steps to ensure a smooth transition from development to production:
- Environment Configuration: Adjust your
.env
file for production settings, including database configurations and application key. - Composer Install: Run
composer install --optimize-autoloader --no-dev
to install dependencies and optimize the autoloader for production. - Artisan Commands: Execute
php artisan config:cache
andphp artisan route:cache
to cache configuration and routes for faster performance. - Migrate Database: Apply migrations to update the production database:
php artisan migrate --force
- Optimize: Optimize your application with:
php artisan optimize --force
Continuous Integration and Deployment Strategies
Implementing continuous integration and deployment (CI/CD) streamlines the deployment process:
- Version Control: Use Git for version control, ensuring a stable codebase.
- CI/CD Services: Integrate with CI/CD services like GitHub Actions or GitLab CI to automate testing and deployment pipelines.
- Automated Tests: Configure CI to run automated tests on each push, ensuring code quality.
- Deployment Scripts: Create deployment scripts that automate the deployment process to production servers.
Tips for Ongoing Maintenance and Updates
Maintaining a Laravel application involves ongoing efforts to keep it secure and up-to-date:
- Regular Backups: Schedule regular backups of your database and files to prevent data loss.
- Security Updates: Stay informed about Laravel and package updates. Apply security patches promptly.
- Performance Monitoring: Implement tools for performance monitoring to identify and address potential bottlenecks.
In the final sections, we’ll guide you through building real-world projects and provide a concluding summary of key points to solidify your expertise.
Building Real-World Projects
Remember, the process of building real-world projects is invaluable for refining your problem-solving abilities and gaining hands-on experience.
Hands-On Projects to Apply Learned Concepts
The best way to solidify your skills is by working on practical, real-world projects. Here are a few project ideas to apply the concepts you’ve learned:
- Task Manager Application: Create a task manager where users can add, edit, and delete tasks. Implement user authentication for personalized task lists.
- Blog Platform: Develop a blog platform with features like user registration, post creation, and commenting. Use the Eloquent ORM for efficient data management.
- E-commerce Store: Build a simple e-commerce store with product listings, shopping cart functionality, and checkout processes. Integrate payment gateways for a complete experience.
- API Integration: Integrate with a third-party API to fetch and display data in your application. This project enhances your understanding of API development.
Showcasing the Practical Application of Laravel
As you work on these projects, consider showcasing them in a portfolio. Highlight the features you’ve implemented, challenges you’ve overcome, and the technologies you’ve integrated.
This not only demonstrates your skills to potential employers but also serves as a valuable reference for your own growth.
Conclusion
In this Ultimate Beginner’s Guide, you will be understanding the basics to exploring advanced topics and building real-world projects.
Let’s recap the key takeaways:
- Foundational Understanding:
- Installed and set up Laravel, grasping its directory structure and MVC architecture.
- Explored Blade templating for dynamic views and leveraged Eloquent ORM for seamless database interactions.
- Introduced to Laravel Artisan commands for streamlined development.
- Mastering Basic Features:
- Explored Laravel routing, middleware for enhanced security, and the built-in authentication system.
- Integrated Laravel with popular frontend frameworks, optimized assets using Laravel Mix, and implemented responsive design.
- Advanced Topics:
- Dived into API development, explored Laravel packages for extended functionality, and received tips for optimizing performance.
- Testing and Debugging:
- Overviewed Laravel’s testing tools and learned debugging techniques for troubleshooting and optimization.
- Deployment and Maintenance:
- Discussed best practices for deploying Laravel applications, continuous integration and deployment strategies, and ongoing maintenance tips.
- Building Real-World Projects:
- Encouraged hands-on projects to apply learned concepts and suggested project ideas such as a task manager, blog platform, e-commerce store, and API integration.
Remember that continuous learning is the key to success in the dynamic field of full-stack web development.
Apply your knowledge to real-world scenarios, stay updated with the evolving ecosystem, and never hesitate to explore new challenges.
Leave a Reply