Mastering relational databases is a pivotal skill that can elevate your proficiency and open doors to endless possibilities. A relational database is essentially a structured collection of data that allows developers to organize and manage information efficiently.
The impact of a solid understanding of relational databases extends far beyond database management itself.
It influences how seamlessly your web applications interact with data, affecting the overall user experience and functionality.
In this article unfolds seven essential insights to help you start strong on your journey to becoming a learn database better.
Quick Links
Insight 1: Understanding the Fundamentals
It’s crucial to establish a solid foundation. In the context of relational databases, this starts with a clear understanding of the fundamentals.
Definition and Purpose
At its core, a relational database is a structured collection of data organized in tables, each consisting of rows and columns.
This tabular structure allows for efficient data retrieval, storage, and management.
Imagine a simple table representing user information:
CREATE TABLE Users (
UserID INT PRIMARY KEY,
UserName VARCHAR(50),
Email VARCHAR(100),
Age INT
);
Here, we’ve created a table named “Users” with columns for UserID, UserName, Email, and Age.
Key Terms: Tables, Rows, Columns, and Relationships
- Tables: Think of them as spreadsheets where data is stored. Each table is dedicated to a specific type of information.
- Rows: Each row in a table represents a record or an entry, containing individual pieces of data.
- Columns: These are the attributes or fields of the data. In the example above, UserID, UserName, Email, and Age are columns.
Understanding these foundational elements lays the groundwork for effective database design and management.
Insight 2: Choosing the Right Database Management System (DBMS)
Now that we’ve laid the groundwork, let’s talk about selecting the right tool for the job—the Database Management System (DBMS). The DBMS is the software that enables you to interact with and manage your relational database.
There are various options available, and choosing the one that aligns with your project requirements is crucial.
Overview of Popular DBMS Options
- MySQL:
- Widely used for its reliability and ease of integration.
- Ideal for projects of varying scales, from small websites to large enterprises.
CREATE DATABASE YourDatabaseName;
- PostgreSQL:
- Known for its advanced features and support for complex queries.
- Suitable for projects where data integrity and accuracy are top priorities.
Example of creating a table in PostgreSQL:
CREATE TABLE YourTableName (
Column1 DataType,
Column2 DataType,
...
);
Factors to Consider When Selecting a DBMS
- Scalability: Consider the potential growth of your project and choose a DBMS that can scale accordingly.
- Complexity of Data: For projects dealing with intricate relationships and extensive data, a robust DBMS like PostgreSQL might be more suitable.
- Community Support: Look for a DBMS with an active community, as it ensures continuous development and reliable support.
Choosing the right DBMS is akin to selecting the right tool for a specific task. Each has its strengths, and understanding them empowers you to make informed decisions.
Insight 3: Database Design Best Practices
With the foundational knowledge in place and the right DBMS at your disposal, it’s time to focus on the art of database design.
This insight revolves around best practices that will shape how your data is organized and accessed.
Normalization and Denormalization Concepts
Normalization is the process of organizing data to reduce redundancy and improve data integrity. It involves breaking down large tables into smaller ones and establishing relationships between them.
Denormalization, on the other hand, involves combining tables to optimize query performance.
Consider a scenario where we have separate tables for Users and Orders:
-- Users Table
CREATE TABLE Users (
UserID INT PRIMARY KEY,
UserName VARCHAR(50),
Email VARCHAR(100),
Age INT
);
-- Orders Table
CREATE TABLE Orders (
OrderID INT PRIMARY KEY,
UserID INT,
OrderDate DATE,
TotalAmount DECIMAL(10, 2),
FOREIGN KEY (UserID) REFERENCES Users(UserID)
);
By linking these tables through the UserID, we establish a relationship while avoiding redundant data.
Structuring Databases for Optimal Performance and Scalability
- Consider Query Patterns: Analyze how data will be queried and structure your tables accordingly.
- Indexing: Strategically use indexes to speed up data retrieval. For instance:
-- Creating an index on the UserID column in the Users table
CREATE INDEX idx_UserID ON Users(UserID);
Effective database design is a balancing act that requires careful consideration of your application’s needs.
Insight 4: SQL Mastery
SQL (Structured Query Language) is the language that empowers developers to interact with relational databases. A mastery of SQL is essential for effective data manipulation, retrieval, and management.
Let’s dive into the key aspects of SQL that will enhance your ability to harness the full potential of relational databases.
Importance of SQL in Interacting with Relational Databases
SQL serves as the bridge between you and your database. Whether you’re retrieving data, updating records, or creating new tables, SQL is the tool that allows you to communicate seamlessly with your database.
Basic and Advanced SQL Commands
- SELECT Statement (Basic):
- Used to retrieve data from one or more tables.
-- Example: Retrieve all columns from the Users table
SELECT * FROM Users;
- UPDATE Statement (Basic):
- Modifies existing data in a table.
-- Example: Update the Age of a user with UserID 1
UPDATE Users SET Age = 25 WHERE UserID = 1;
- JOIN Clause (Advanced):
- Combines rows from two or more tables based on a related column.
-- Example: Retrieve user information along with their order details
SELECT Users.UserName, Orders.OrderDate
FROM Users
JOIN Orders ON Users.UserID = Orders.UserID;
Mastering these fundamental and advanced SQL commands empowers you to shape and manipulate data according to your application’s requirements.
Insight 5: Indexing Strategies
In the intricate world of relational databases, indexing plays a crucial role in optimizing data retrieval speed and overall performance.
Understanding and implementing effective indexing strategies can significantly enhance the efficiency of your database operations.
Let’s unravel the significance of indexing and explore different strategies for harnessing its power.
Significance of Indexing in Enhancing Database Performance
Think of an index as a roadmap for your database engine. It allows it to quickly locate specific rows within a table, minimizing the time required for queries.
Without proper indexing, your database might resemble a vast library without a card catalog—a bit chaotic and time-consuming to navigate.
Different Indexing Strategies and When to Use Them
- Single-Column Index: Created on a single column, ideal for scenarios where queries often involve a specific column.
-- Example: Creating an index on the UserName column in the Users table
CREATE INDEX idx_UserName ON Users(UserName);
- Composite Index: Involves multiple columns and is beneficial when queries filter based on a combination of these columns.
-- Example: Creating a composite index on the UserID and OrderDate columns in the Orders table
CREATE INDEX idx_UserOrder ON Orders(UserID, OrderDate);
Understanding the nature of your queries and the structure of your data is key to choosing the right indexing strategy.
Insight 6: Security Measures for Databases
In the ever-evolving landscape of web development, ensuring the security of your databases is paramount. Aspiring full-stack web developers must grasp the fundamentals of safeguarding sensitive data from unauthorized access and potential threats.
Let’s delve into the security measures that will fortify the integrity of your relational databases.
Protecting Sensitive Data Through Access Controls
- User Permissions: Assign specific permissions to users based on their roles (e.g., read-only, read-write).
-- Example: Granting read-only access to a user on the Users table
GRANT SELECT ON Users TO YourReadOnlyUser;
- Authentication Mechanisms: Implement secure authentication methods, such as hashed passwords, to ensure only authorized users can access the database.
Implementing Encryption and Other Security Best Practices
- Data Encryption: Encrypt sensitive data, such as passwords and confidential information, to protect it from unauthorized access.
-- Example: Encrypting a column with sensitive information
ALTER TABLE Users
MODIFY COLUMN Password VARBINARY(256) ENCRYPTED WITH 'your_encryption_algorithm';
- Regular Auditing: Set up regular audits to track database activities and identify potential security breaches.
Prioritizing database security is not only a best practice but a responsibility that comes with handling user data.
Insight 7: Troubleshooting and Optimization
Congratulations on reaching the final insight!
As you continue on your journey to master relational databases, it’s crucial to equip yourself with the skills to troubleshoot common issues and optimize database performance.
Let’s explore the strategies that will empower you to overcome challenges and fine-tune your database for seamless functionality.
Common Challenges in Database Development and How to Overcome Them
- Query Performance Issues: Identify and optimize slow-performing queries by analyzing their execution plans.
-- Example: Analyzing the execution plan for a query
EXPLAIN SELECT * FROM Users WHERE Age > 30;
- Data Integrity Problems: Implement constraints and validations to maintain data accuracy and integrity.
-- Example: Adding a constraint to ensure Age is always a positive integer
ALTER TABLE Users
ADD CONSTRAINT CHK_PositiveAge CHECK (Age > 0);
Strategies for Optimizing Database Performance and Resolving Issues
- Regular Maintenance: Schedule routine maintenance tasks, such as index rebuilding and statistics updating, to keep your database in top shape.
- Database Monitoring: Utilize monitoring tools to keep a close eye on database performance metrics and identify potential issues before they escalate.
By honing your troubleshooting and optimization skills, you’ll be well-equipped to tackle the intricacies of database development.
Conclusion
Congratulations, aspiring full-stack web developers! You’ve navigated through the essential insights of mastering relational databases, laying a robust foundation for your journey in the dynamic world of web development.
Let’s recap the key takeaways and encourage you to forge ahead with newfound skills and confidence.
Recap of the Seven Essential Insights
- Understanding the Fundamentals:
- Defined the basics of relational databases, including tables, rows, columns, and their importance in data organization.
- Choosing the Right Database Management System (DBMS):
- Explored popular DBMS options and factors to consider when selecting the most suitable one for your projects.
- Database Design Best Practices:
- Delved into normalization, denormalization, and effective structuring of databases for optimal performance.
- SQL Mastery:
- Emphasized the significance of SQL in interacting with databases and provided examples of basic and advanced SQL commands.
- Indexing Strategies:
- Explored the importance of indexing in enhancing database performance and discussed different strategies for optimal results.
- Security Measures for Databases:
- Discussed essential security measures, including user permissions, authentication, encryption, and auditing.
- Troubleshooting and Optimization:
- Equipped you with strategies to overcome common challenges in database development and optimize performance.
Encouragement for Aspiring Full-Stack Web Developers
Embrace challenges as opportunities to learn and refine your skills. Stay curious, explore new technologies, and remain committed to honing your craft.
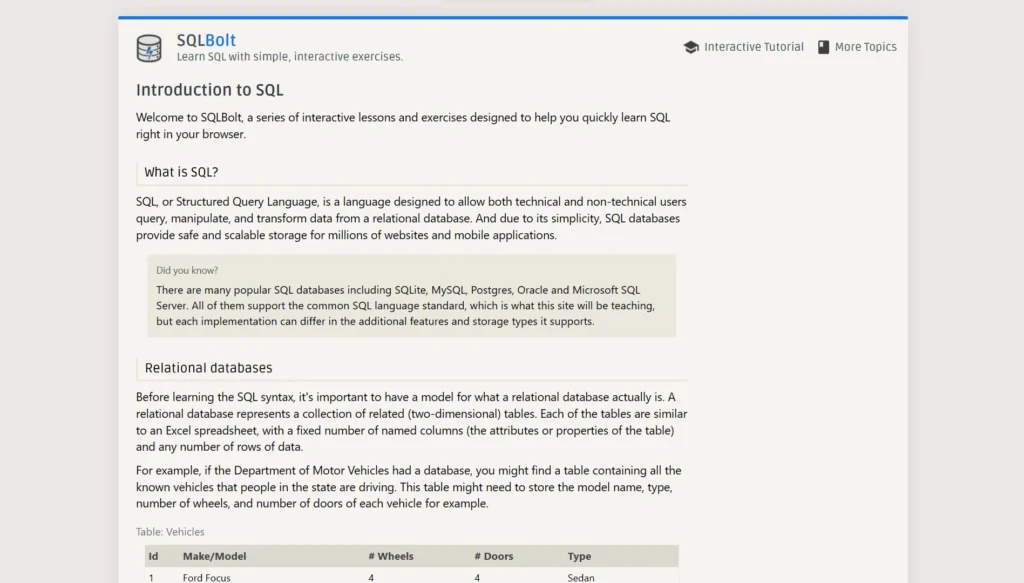
Your understanding of relational databases is not just a skill—it’s a superpower that will set you apart in the competitive landscape of web development.
Now, go forth and build incredible, data-driven web applications with confidence!
Leave a Reply