NoSQL databases have emerged as a game-changer in handling the complexities of modern web applications.
Unlike traditional relational databases, NoSQL databases offer flexibility in managing unstructured data, providing scalability, and accommodating the dynamic nature of web development.
A full-stack web developer navigates both the front-end and back-end realms of a web application. The efficiency of a full-stack developer directly influences the seamless functioning of the entire system.
In this article, we will explore nine proven strategies that empower full-stack developers to optimize their approach to NoSQL databases.
These strategies range from choosing the right database to implementing advanced techniques like horizontal scaling and asynchronous programming.
Quick Links
Understanding the NoSQL Landscape
Definition and Types of NoSQL Databases
NoSQL, or “Not Only SQL,” encompasses a variety of database management systems that depart from the rigid structures of traditional relational databases.
Types of NoSQL databases include document-oriented (e.g., MongoDB), key-value stores (e.g., Redis), wide-column stores (e.g., Cassandra), and graph databases (e.g., Neo4j).
Each type caters to specific use cases, offering flexibility in handling diverse data structures.
Comparison with Traditional Relational Databases
Contrasting NoSQL with traditional relational databases reveals the strengths and weaknesses of each approach.
While relational databases excel in maintaining structured data through tables and predefined schemas, NoSQL databases embrace a more dynamic and scalable approach, accommodating unstructured and evolving data.
Real-World Applications and Use Cases
NoSQL databases find applications in various domains, from social media platforms to e-commerce websites.
MongoDB, for instance, shines in document storage for content management systems, while Cassandra excels in handling large-scale data across distributed environments.
Real-world use cases will illustrate how different NoSQL databases address specific challenges, providing valuable insights for full-stack developers.
Now that we’ve laid the groundwork, our journey continues with strategies for choosing the right NoSQL database, a crucial decision that significantly impacts overall efficiency.
Strategy 1: Choosing the Right NoSQL Database
Selecting the appropriate NoSQL database sets the foundation for a robust and efficient web application.
In this section, we’ll delve into the key considerations for making this decision, explore popular NoSQL databases such as MongoDB, Cassandra, and Redis, and examine real-world case studies illustrating the impact of the chosen database on overall efficiency.
Considerations for Selecting the Appropriate NoSQL Database
Before diving into the specifics of individual databases, it’s essential to consider factors such as data structure, scalability requirements, and query patterns.
Understanding the nature of your data and the anticipated workload will guide you in choosing a NoSQL database that aligns with your application’s unique needs.
Overview of Popular NoSQL Databases
MongoDB
MongoDB, a leading document-oriented database, excels in flexibility and scalability. Its JSON-like documents make it ideal for storing and querying diverse data types.
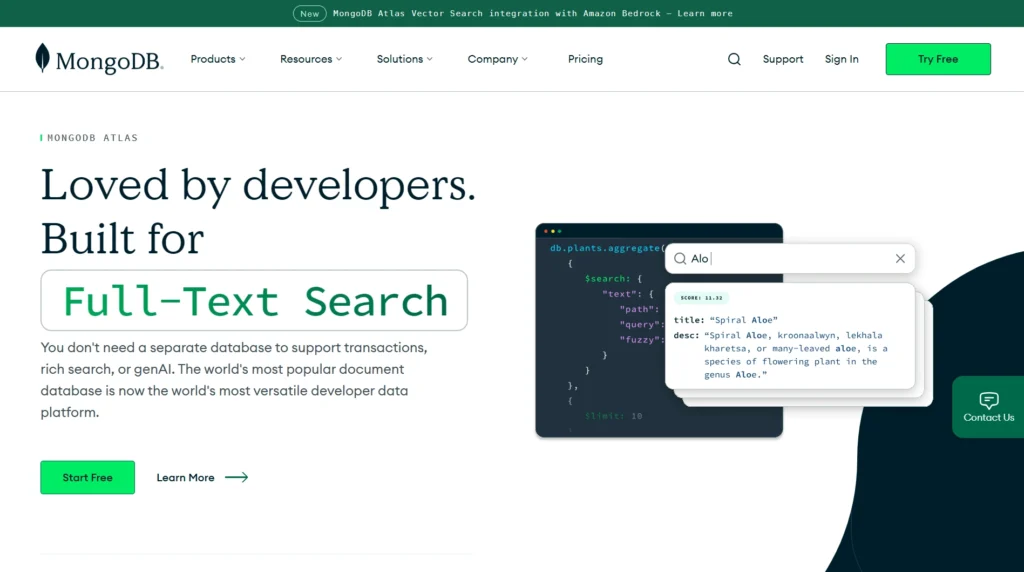
Sample code snippet for inserting a document:
db.collection('users').insertOne({
name: 'John Doe',
age: 30,
email: 'john@example.com'
});
Cassandra
Cassandra, a wide-column store, thrives in distributed environments and handles massive amounts of data. Example of creating a keyspace and table:
CREATE KEYSPACE mykeyspace WITH replication = {'class': 'SimpleStrategy', 'replication_factor': 1};
CREATE TABLE mykeyspace.mytable (
id UUID PRIMARY KEY,
name TEXT,
age INT
);
Redis
Redis, a key-value store, specializes in high-performance data storage.
Simple example of setting and retrieving a key-value pair:
import redis
r = redis.StrictRedis(host='localhost', port=6379, db=0)
r.set('mykey', 'Hello, Redis!')
value = r.get('mykey')
Case Studies Illustrating the Impact of Database Choice on Efficiency
Real-world examples will illuminate the consequences of database choices. For instance, a social media platform might benefit from MongoDB’s agility in handling user-generated content, while an analytics-heavy application could leverage Cassandra’s distributed architecture for seamless scalability.
By understanding the strengths and use cases of different NoSQL databases, full-stack developers can make informed decisions that lay the groundwork for an efficient and scalable web application.
The journey continues with data modeling for NoSQL, a crucial aspect of optimizing efficiency.
Strategy 2: Data Modeling for NoSQL
Efficiency in NoSQL databases hinges on effective data modeling. In this section, we’ll explore the importance of a flexible schema design, techniques for crafting optimal data models, and case studies showcasing how tailored data structures enhance performance in specific scenarios.
Importance of Flexible Schema Design
Unlike rigid schemas in traditional databases, NoSQL databases thrive on flexibility. Embracing a dynamic schema design allows for agile adaptation to evolving application requirements.
This flexibility proves invaluable in scenarios where data structures are subject to frequent changes, providing a foundation for efficient development cycles.
Techniques for Effective Data Modeling in NoSQL Databases
Document-Oriented Databases (e.g., MongoDB)
Utilize embedded documents and arrays to represent complex relationships within a single document. This reduces the need for complex joins and enhances query performance.
Example of a nested document in MongoDB:
{
"_id": 1,
"title": "Blog Post",
"author": {
"name": "John Doe",
"age": 30,
"email": "john@example.com"
},
"comments": [
{
"text": "Great post!",
"user": "Alice"
},
{
"text": "I have a question.",
"user": "Bob"
}
]
}
Wide-Column Stores (e.g., Cassandra)
Leverage denormalization and duplicate data when necessary to optimize read performance. Design tables based on query patterns to minimize the need for complex joins.
Example of a denormalized table in Cassandra:
CREATE TABLE user_activity (
user_id UUID,
activity_date DATE,
activity_type TEXT,
details TEXT,
PRIMARY KEY (user_id, activity_date)
) WITH CLUSTERING ORDER BY (activity_date DESC);
Case Studies Showcasing Optimized Data Structures for Specific Scenarios
Examining real-world scenarios, we’ll illustrate how thoughtful data modeling contributes to efficiency.
For instance, a messaging application might benefit from MongoDB’s ability to store chat messages as nested documents, simplifying retrieval and updates.
As we navigate through data modeling strategies, the next leg of our journey explores indexing and query optimization in NoSQL databases, vital for achieving peak performance in full-stack applications.
Strategy 3: Indexing and Query Optimization
Efficiency in NoSQL databases goes hand in hand with effective indexing and query optimization.
In this section, we’ll delve into the nuances of indexing, techniques for optimizing queries, and real-world examples demonstrating how strategic indexing enhances performance in full-stack applications.
Understanding Indexing in NoSQL Databases
Indexes play a pivotal role in speeding up data retrieval operations. In NoSQL databases, creating indexes on specific fields significantly improves query performance.
Understanding the types of indexes available for each database type is crucial for making informed decisions.
Techniques for Optimizing Queries for Improved Performance
MongoDB
In MongoDB, the choice of indexes depends on the nature of queries. For example, creating an index on a field used in a query condition can drastically accelerate the search process.
Example of creating an index in MongoDB:
db.collection('products').createIndex({ name: 1 });
Cassandra
Cassandra utilizes compound keys for efficient querying. Designing tables with appropriate primary and clustering keys can eliminate the need for full-table scans.
Example of creating a table with a compound primary key in Cassandra:
CREATE TABLE user_activity (
user_id UUID,
activity_date DATE,
activity_type TEXT,
details TEXT,
PRIMARY KEY (user_id, activity_date)
) WITH CLUSTERING ORDER BY (activity_date DESC);
Real-World Examples of Query Optimization in Full-Stack Applications
Explore scenarios where strategic indexing and query optimization make a tangible difference.
For instance, in an e-commerce platform, optimizing queries for product searches can significantly reduce response times, leading to a smoother user experience.
As we delve into the intricacies of indexing and query optimization, the next strategy on our journey focuses on horizontal scaling for enhanced performance in web development.
Stay tuned for insights into the benefits of horizontal scaling and effective strategies for implementation.
Strategy 4: Horizontal Scaling for Performance
Efficiency in web development often demands the ability to scale seamlessly with increasing demands.
In this section, we’ll explore horizontal scaling, its benefits, strategies for implementation through sharding and partitioning, and real-world case studies showcasing successful horizontal scaling in web development.
Exploring Horizontal Scaling and Its Benefits
Horizontal scaling involves distributing data across multiple servers to handle increased load.
Unlike vertical scaling, which involves upgrading a single server’s resources, horizontal scaling offers a more cost-effective and flexible solution to accommodate growing user bases and data volumes.
Implementing Sharding and Partitioning Strategies
Sharding
Sharding involves dividing a large dataset into smaller, more manageable pieces called shards, each residing on a separate server.
This allows for parallel processing of queries and distributes the load evenly.
Example of sharding in MongoDB:
sh.shardCollection("mydb.mycollection", { "shardkey": 1 });
Partitioning
Partitioning involves dividing a table into smaller subsets known as partitions based on specific criteria.
This ensures that queries only access the relevant partitions, reducing the overall query time.
Example of partitioning in Cassandra:
CREATE TABLE user_activity (
user_id UUID,
activity_date DATE,
activity_type TEXT,
details TEXT,
PRIMARY KEY ((user_id, activity_date), activity_type)
) WITH CLUSTERING ORDER BY (activity_date DESC);
Case Studies of Successful Horizontal Scaling in Web Development
Explore instances where horizontal scaling proved instrumental in handling increased workloads.
For instance, a social media platform experiencing a surge in user activity can seamlessly distribute the load using sharding, ensuring a consistent and responsive user experience.
As we unravel the strategies for enhancing efficiency, the next focus will be on caching strategies—a crucial element in optimizing performance in full-stack web development.
Stay tuned for insights into the importance of caching and practical examples of its implementation.
Strategy 5: Caching Strategies
Efficiency in full-stack web development often relies on the strategic use of caching.
In this section, we’ll delve into the importance of caching, explore techniques for implementing caching strategies with NoSQL databases, and examine real-world examples highlighting how caching enhances efficiency in various applications.
Importance of Caching in Full-Stack Web Development
Caching involves storing frequently accessed data in a temporary storage location, reducing the need to repeatedly fetch the same data from the database.
This results in faster response times, lower latency, and a more responsive user experience.
Implementing Caching Strategies with NoSQL Databases
Redis as a Cache
Redis, a versatile key-value store, is often employed as a caching solution. By storing frequently accessed data in Redis, developers can accelerate read operations and alleviate the load on the primary database.
Example of caching with Redis in Node.js:
const redis = require('redis');
const client = redis.createClient();
// Check if data is in cache
client.get('cachedData', (err, data) => {
if (err) throw err;
if (data !== null) {
// Data found in cache, use it
console.log('Data from cache:', data);
} else {
// Fetch data from the primary database
fetchDataFromDatabase((result) => {
// Store data in cache for future use
client.set('cachedData', result);
console.log('Data from database:', result);
});
}
});
Examples of How Caching Enhances Efficiency in Real-World Applications
Explore scenarios where caching proves instrumental in improving efficiency. For instance, an e-commerce website can cache product information, ensuring faster page loads and a seamless shopping experience.
Real-world examples will illuminate how caching strategies contribute to overall system performance.
As we continue our exploration of efficiency strategies, the next focus will be on asynchronous programming with NoSQL databases.
Stay tuned for insights into the advantages of asynchronous patterns and their integration with NoSQL, accompanied by practical use cases demonstrating their impact on efficiency.
Strategy 6: Asynchronous Programming with NoSQL
Efficiency in full-stack web development often involves leveraging asynchronous programming.
In this section, we’ll provide an overview of asynchronous programming, explore its advantages, and discuss the integration of asynchronous patterns with NoSQL databases.
Real-world use cases will illustrate the tangible impact of asynchronous programming on overall system efficiency.
Overview of Asynchronous Programming and Its Advantages
Asynchronous programming allows tasks to run independently, freeing up resources to handle other operations concurrently.
This approach is particularly beneficial in scenarios where tasks involve waiting for external resources, such as database queries, file I/O, or network requests.
Integrating Asynchronous Patterns with NoSQL Databases
Node.js and MongoDB Example
In Node.js, which excels in handling asynchronous operations, integrating with MongoDB using asynchronous patterns can enhance overall system responsiveness.
Example of asynchronous MongoDB query in Node.js:
const MongoClient = require('mongodb').MongoClient;
// Connect to MongoDB
MongoClient.connect('mongodb://localhost:27017/mydb', (err, client) => {
if (err) throw err;
// Asynchronously query the database
const db = client.db('mydb');
db.collection('mycollection').find({}).toArray((err, result) => {
if (err) throw err;
// Process the result
console.log('Query result:', result);
// Close the database connection
client.close();
});
});
Use Cases Demonstrating the Impact of Asynchronous Programming on Efficiency
Explore instances where asynchronous programming significantly improves system efficiency.
For instance, in a real-time chat application, asynchronous handling of messages allows the server to respond promptly to user interactions, creating a seamless and responsive user experience.
As our exploration of efficiency strategies continues, the next focus will be on error handling and resilience.
We’ll delve into building robust error-handling mechanisms in full-stack applications and explore strategies for ensuring resilience in the face of database failures.
Stay tuned for insights into learning from real-world scenarios where effective error handling made a significant difference.
Strategy 7: Error Handling and Resilience
In the dynamic landscape of full-stack web development, robust error handling and resilience are paramount.
In this section, we’ll explore the importance of building strong error-handling mechanisms, strategies for ensuring resilience in the face of database failures, and real-world scenarios where effective error handling has made a significant difference.
Building Robust Error-Handling Mechanisms in Full-Stack Applications
Effective error handling involves anticipating potential issues, gracefully handling errors, and providing meaningful feedback to users and developers.
Logging, proper status codes, and informative error messages play a crucial role in diagnosing and resolving issues promptly.
Strategies for Ensuring Resilience in the Face of Database Failures
Databases are a critical component of web applications, and their occasional failures are inevitable.
Implementing strategies like retry mechanisms, fallback mechanisms, and failover mechanisms ensures that applications remain operational even when faced with temporary database outages.
Example of retry mechanism in Node.js using MongoDB:
const MongoClient = require('mongodb').MongoClient;
// Function to connect to MongoDB with retry
async function connectWithRetry(url, options, retryCount) {
try {
const client = await MongoClient.connect(url, options);
console.log('Connected to MongoDB');
return client;
} catch (err) {
console.error('Error connecting to MongoDB:', err.message);
if (retryCount > 0) {
console.log(`Retrying connection (attempts left: ${retryCount})...`);
await new Promise(resolve => setTimeout(resolve, 5000)); // Wait for 5 seconds before retrying
return connectWithRetry(url, options, retryCount - 1);
} else {
throw err; // Propagate the error if retries are exhausted
}
}
}
// Usage
const client = await connectWithRetry('mongodb://localhost:27017/mydb', { useNewUrlParser: true }, 3);
Learning from Real-World Scenarios Where Error Handling Made a Difference
Explore scenarios where effective error handling and resilience proved crucial. For instance, during a sudden surge in user activity, a well-designed error-handling mechanism can prevent service disruptions and maintain a positive user experience.
As we progress through our strategies for boosting efficiency, the next focus will be on security best practices.
We’ll address the critical considerations in securing NoSQL databases, implementing authentication and authorization measures, and examining case studies that highlight the consequences of inadequate security practices.
Stay tuned for insights into fortifying your web applications against security threats.
Strategy 8: Security Best Practices
Security is a cornerstone in the development of robust web applications, and NoSQL databases are no exception.
In this section, we’ll address the critical considerations in securing NoSQL databases, implementing authentication and authorization measures, and delve into case studies that underscore the consequences of inadequate security practices.
Addressing Security Considerations in NoSQL Databases
Securing NoSQL databases involves safeguarding data against unauthorized access, ensuring data integrity, and protecting against potential vulnerabilities.
Key considerations include encryption of data in transit and at rest, access control mechanisms, and regular security audits.
Implementing Authentication and Authorization Measures
MongoDB Authentication Example
MongoDB, like many NoSQL databases, supports authentication to control access to databases and collections.
Example of creating a user with specific roles:
use admin;
db.createUser({
user: 'myAdminUser',
pwd: 'myAdminPassword',
roles: [
{ role: 'userAdminAnyDatabase', db: 'admin' },
{ role: 'readWriteAnyDatabase', db: 'admin' },
{ role: 'dbAdminAnyDatabase', db: 'admin' }
]
});
Case Studies Highlighting the Consequences of Inadequate Security Practices
Explore real-world examples where inadequate security practices led to data breaches and compromises.
Learning from these cases underscores the importance of implementing robust security measures.
For instance, an unsecured NoSQL database could expose sensitive user information, resulting in legal and reputational repercussions.
As our exploration of efficiency strategies continues, the next focus will be on continuous learning and staying abreast of NoSQL trends.
We’ll delve into the ever-evolving landscape of NoSQL technologies, provide resources for staying updated on the latest developments, and emphasize the importance of continuous learning for aspiring full-stack developers.
Stay tuned for insights into keeping your skills sharp in the rapidly changing world of web development.
Strategy 9: Continuous Learning and Keeping Abreast of NoSQL Trends
In the dynamic realm of technology, continuous learning is not just a practice but a necessity.
In this section, we’ll explore the ever-evolving landscape of NoSQL technologies, provide resources for staying updated on the latest developments, and emphasize the importance of continuous learning for aspiring full-stack developers.
The Ever-Evolving Landscape of NoSQL Technologies
NoSQL technologies undergo constant evolution with the introduction of new databases, features, and optimization techniques.
Staying informed about emerging trends ensures that developers are well-equipped to make informed decisions, adapt to changes, and harness the latest advancements for enhanced efficiency.
Resources for Staying Updated on the Latest Developments
Online Communities and Forums
Participating in online communities and forums, such as Stack Overflow, Reddit’s programming communities, and NoSQL-specific forums, provides avenues for seeking advice, sharing experiences, and staying informed about recent developments.
Conferences and Meetups
Attending conferences, both virtual and in-person, offers opportunities to connect with industry experts, gain insights from keynote speakers, and explore hands-on workshops focused on the latest NoSQL trends.
Documentation and Official Blogs
Regularly reviewing documentation and official blogs of NoSQL databases keeps developers abreast of new features, best practices, and updates. This direct source of information ensures accuracy and reliability.
The Importance of Continuous Learning for Aspiring Full-Stack Developers
Web development is a field that thrives on innovation, and staying stagnant is not an option.
Continuous learning empowers full-stack developers to adapt to changing requirements, embrace new technologies, and maintain a competitive edge in the job market.
It’s a journey of growth that extends beyond mastering a specific technology stack to cultivating a mindset of curiosity and adaptability.
As we approach the conclusion of our exploration, let’s recap the nine proven strategies for boosting efficiency and empowering full-stack web developers to navigate the complexities of NoSQL databases.
In the next section, we’ll provide encouragement for aspiring developers to embark on their NoSQL journey and a call to action for implementing these strategies in real-world projects.
Stay tuned for the concluding thoughts that encapsulate the essence of efficient NoSQL development.
Conclusion
The strategies outlined here are not just tools; they are guiding principles on your journey to becoming an efficient and adept developer.
The true essence of these strategies lies in their application. Take the knowledge gained and infuse it into your real-world projects. Experiment, iterate, and learn from each implementation.
Whether you’re building a personal project, contributing to open-source endeavors, or working in a professional setting, the application of these strategies will undoubtedly elevate the efficiency of your web development endeavors.
Happy coding!
Leave a Reply