If you’re aspiring to become a full-stack web developer, Python is a fantastic language to add to your toolkit.
In this comprehensive guide, we’ll walk you through the basics of Python and show you how it fits seamlessly into both the frontend and backend aspects of web development.
Quick Links
Brief Overview of Python in Full-Stack Development
Python has gained immense popularity for its readability, versatility, and a rich ecosystem of libraries and frameworks.
As a full-stack web developer, you’ll find Python to be an excellent choice for both server-side and client-side development.
Python’s simplicity and clean syntax make it an ideal language for building robust web applications.
It’s not just about the code; it promotes a structured and organized approach to development, which is crucial when working on complex full-stack projects.
Now, let’s dive into the basics of getting started with Python.
Getting Started
Installing Python
Before we continue on our full-stack journey, let’s make sure Python is set up on your machine.
You can download the latest version from the official Python website.
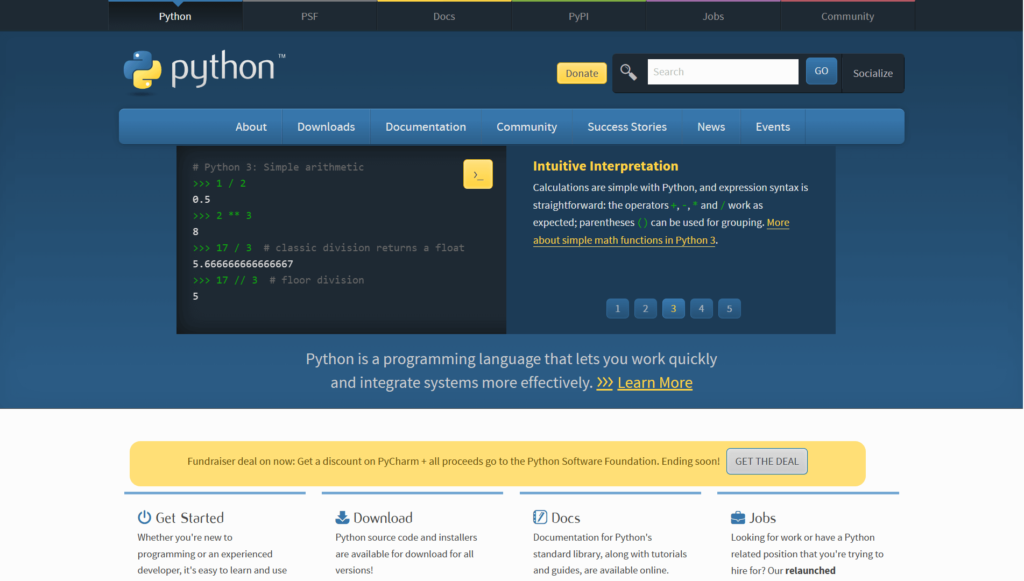
Once installed, you can check the version by opening a terminal or command prompt and typing:
python --version
Setting Up a Virtual Environment
To keep your project dependencies isolated, it’s good practice to use virtual environments.
Create one using the following commands:
# On Windows
python -m venv venv
# On macOS/Linux
python3 -m venv venv
Activate the virtual environment:
# On Windows
venv\Scripts\activate
# On macOS/Linux
source venv/bin/activate
Now, you’re ready to start coding in a clean environment. Next, let’s explore Python versions and package management using pip.
Understanding Python Versions and Package Management (pip)
Python has two major versions: Python 2 and Python 3. As of now, Python 3 is the recommended version, as Python 2 is no longer supported.
To install packages, we use pip
, the Python package installer.
For example, to install a package like Flask (a popular web framework), use:
pip install Flask
With Python set up, and your virtual environment ready, we’re prepared to delve into the basics of Python for full-stack development.
In the next section, we’ll cover fundamental concepts like variables, data types, control flow, and more.
Python Basics for Full-Stack Developers
Now that you’ve got Python installed and your virtual environment set up, let’s start exploring the foundational concepts that will serve as the building blocks for your full-stack development journey.
Variables and Data Types
In Python, variables are used to store and manage data. Let’s create a simple variable:
message = "Hello, Full-Stack Developer!"
print(message)
In this example, message
is a variable storing a string. It is dynamically typed, meaning you don’t need to explicitly declare the variable’s type.
Data types in Python include integers, floats, strings, lists, and more. Here’s a quick example:
age = 25
height = 1.75
name = "John"
skills = ["Python", "HTML", "CSS"]
Control Flow and Loops
Control flow structures, like if
, else
, and elif
, help manage the flow of your program. For instance:
x = 10
if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than 5")
Loops, such as for
and while
, are crucial for iterating over data:
# For loop
for skill in skills:
print("I know:", skill)
# While loop
counter = 0
while counter < 5:
print("Counting:", counter)
counter += 1
Functions and Modules
Functions allow you to encapsulate code for reuse. Here’s a simple function:
def greet(name):
print("Hello, " + name + "!")
# Call the function
greet("Alice")
Modules are files containing Python code that can be imported into other files. For example:
# math_operations.py
def add(x, y):
return x + y
# main.py
from math_operations import add
result = add(3, 5)
print("Result:", result)
Modules are files containing Python code that can be imported into other files. For example:
# math_operations.py
def add(x, y):
return x + y
# main.py
from math_operations import add
result = add(3, 5)
print("Result:", result)
Modules are files containing Python code that can be imported into other files. For example:
# math_operations.py
def add(x, y):
return x + y
# main.py
from math_operations import add
result = add(3, 5)
print("Result:", result)
Exception Handling
Handling errors gracefully is crucial. Use try
, except
blocks:
try:
result = 10 / 0
except ZeroDivisionError:
print("Error: Cannot divide by zero.")
With these Python basics under your belt, you’re well-equipped to move on to the exciting world of full-stack web development.
In the next section, we’ll explore Python’s role in the backend, building a simple web application.
Python in the Backend
With a solid grasp of Python basics, it’s time to explore its role in the backend of web development. It offers powerful frameworks like Django and Flask that simplify the process of building robust web applications.
Introduction to Web Frameworks (Django, Flask)
Web frameworks provide a structure for developing web applications. Django is a high-level, batteries-included framework, while Flask is a lightweight, micro-framework.
Let’s explore a basic example using Flask:
# app.py (Flask example)
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return 'Hello, Flask World!'
if __name__ == '__main__':
app.run(debug=True)
In this example, we create a web application with a single route (“/”) that returns a greeting when accessed.
Building a Simple Web Application
Let’s extend our Flask example to include routing, templates, and form handling:
# app.py (Extended Flask example)
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route('/')
def home():
return 'Hello, Flask World!'
@app.route('/greet/<name>')
def greet(name):
return render_template('greet.html', name=name)
@app.route('/submit', methods=['POST'])
def submit():
if request.method == 'POST':
return 'Form submitted successfully!'
if __name__ == '__main__':
app.run(debug=True)
This extended example includes a dynamic route (/greet/<name>
) and a simple form submission route (/submit
).
By understanding these basic concepts, you’re on your way to building dynamic web applications with Python in the backend.
In the next section, we’ll explore how the language seamlessly integrates with frontend technologies, allowing you to create a cohesive full-stack experience.
Integrating Frontend with Python
Python’s versatility extends beyond the backend; it also plays a crucial role in integrating with frontend technologies like HTML, CSS, and JavaScript.
Let’s explore how the language seamlessly works with these technologies to create dynamic and interactive user interfaces.
Overview of Frontend Technologies (HTML, CSS, JavaScript)
Before diving into integration, let’s briefly revisit the frontend technologies you’ll encounter in full-stack development:
- HTML (Hypertext Markup Language): Defines the structure of a web page.
- CSS (Cascading Style Sheets): Styles and enhances the visual presentation of HTML.
- JavaScript: Adds interactivity and dynamic behavior to web pages.
Template Engines and Rendering Dynamic Content
Python’s backend frameworks often use template engines to dynamically generate HTML content. In Flask, for example, you can use Jinja2.
Here’s a simple example:
# greet.html (Jinja2 template)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Greeting Page</title>
</head>
<body>
<h1>Hello, {{ name }}!</h1>
</body>
</html>
In this example, the {{ name }}
syntax is a placeholder that will be dynamically replaced with the provided name when the page is rendered.
AJAX for Asynchronous Communication
Asynchronous JavaScript and XML (AJAX) enables seamless communication between the frontend and backend without reloading the entire page. You can use the language to create APIs on the backend, and JavaScript to make asynchronous requests.
Here’s a simplified example using Flask and JavaScript:
# app.py (Flask API endpoint)
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/data')
def get_data():
data = {'message': 'Hello from the backend!'}
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
In your JavaScript file:
// script.js
fetch('/api/data')
.then(response => response.json())
.then(data => console.log(data.message))
.catch(error => console.error('Error:', error));
This example demonstrates how Python on the backend can serve data to the frontend asynchronously.
By mastering the integration of Python with frontend technologies, you’ll be able to create seamless and interactive user experiences.
In the upcoming sections, we’ll delve into database interaction, RESTful APIs, testing, and deployment to complete your full-stack journey.
Database Interaction with Python
As a full-stack web developer, understanding how to interact with databases is essential for storing and retrieving data.
Python makes this process smooth with its support for various database types and powerful Object-Relational Mapping (ORM) tools.
Let’s explore the fundamentals of database interaction.
Connecting to Databases (SQL and NoSQL)
Python supports both SQL (Structured Query Language) and NoSQL databases. Libraries like SQLAlchemy provide a unified interface for working with different database types.
Connecting to an SQLite Database (SQL)
# Using SQLAlchemy with SQLite
from sqlalchemy import create_engine, Column, Integer, String, text
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# Define the database model
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
username = Column(String, unique=True)
# Create an SQLite database in memory
engine = create_engine('sqlite:///:memory:', echo=True)
# Create tables
Base.metadata.create_all(bind=engine)
# Create a session to interact with the database
Session = sessionmaker(bind=engine)
session = Session()
# Add a user to the database
new_user = User(username='JohnDoe')
session.add(new_user)
session.commit()
# Query the database
result = session.query(User).filter_by(username='JohnDoe').first()
print("User:", result.username)
Connecting to a MongoDB Database (NoSQL):
# Using pymongo with MongoDB
import pymongo
# Connect to MongoDB
client = pymongo.MongoClient("mongodb://localhost:27017/")
database = client["mydatabase"]
collection = database["users"]
# Insert a document
user_data = {"username": "JaneDoe", "email": "jane@example.com"}
user_id = collection.insert_one(user_data).inserted_id
# Query the collection
user = collection.find_one({"_id": user_id})
print("User:", user)
ORM (Object-Relational Mapping) with Django
Django, a high-level web framework, comes with its built-in ORM. Here’s a quick example:
# models.py (Django ORM example)
from django.db import models
class User(models.Model):
username = models.CharField(max_length=50, unique=True)
# Create and save a user
new_user = User(username='DjangoUser')
new_user.save()
# Query the database
retrieved_user = User.objects.get(username='DjangoUser')
print("User:", retrieved_user.username)
Understanding how to connect, query, and manipulate data in databases is a crucial skill for any full-stack developer.
In the next section, we’ll explore how to build RESTful APIs with Python, enabling communication between the frontend and backend of your web applications.
RESTful APIs with Python
RESTful APIs (Representational State Transfer) serve as a bridge between the frontend and backend, allowing seamless communication and data exchange.
Python, with its web frameworks like Django Rest Framework (DRF) and Flask-RESTful, makes it straightforward to build and consume RESTful APIs.
Building APIs with Django Rest Framework
Django Rest Framework simplifies the process of creating APIs in Django. Here’s a basic example:
# serializers.py (Django Rest Framework)
from rest_framework import serializers
class UserSerializer(serializers.Serializer):
id = serializers.IntegerField()
username = serializers.CharField(max_length=50)
email = serializers.EmailField()
# views.py (Django Rest Framework)
from rest_framework.views import APIView
from rest_framework.response import Response
from rest_framework import status
class UserAPIView(APIView):
def get(self, request, *args, **kwargs):
user_data = {"id": 1, "username": "APIUser", "email": "api@example.com"}
serializer = UserSerializer(user_data)
return Response(serializer.data, status=status.HTTP_200_OK)
In this example, we create a simple API endpoint that returns user data in JSON format.
Consuming APIs in the Frontend
Now, let’s see how you can consume this API in the frontend using JavaScript. Using the Fetch API:
// script.js (Frontend API consumption)
fetch('/api/user')
.then(response => response.json())
.then(data => console.log("User:", data))
.catch(error => console.error('Error:', error));
This JavaScript code makes an asynchronous request to the API endpoint we created and logs the user data to the console.
By mastering the creation and consumption of RESTful APIs, you can establish a smooth flow of data between your frontend and backend.
In the upcoming sections, we’ll explore testing and debugging in Python, as well as the crucial aspects of deployment and scaling your full-stack applications.
Testing and Debugging
Ensuring the reliability and robustness of your code is a critical aspect of full-stack web development. It provides powerful tools and frameworks for testing and debugging, allowing you to catch and fix issues before they impact your users.
Writing Unit Tests
Unit testing involves testing individual units or components of your code to ensure they work as expected. Python’s built-in unittest
module and third-party libraries like pytest
make it easy to write and execute tests.
Here’s a simple example using unittest
:
# test_calculator.py (Unit test example)
import unittest
def add(x, y):
return x + y
class TestCalculator(unittest.TestCase):
def test_addition(self):
result = add(3, 5)
self.assertEqual(result, 8)
if __name__ == '__main__':
unittest.main()
Run the test using:
python test_calculator.py
Debugging Tools and Techniques
Python offers effective debugging tools to identify and fix issues in your code. The pdb
(Python Debugger) module is a built-in tool that allows you to set breakpoints, inspect variables, and step through your code.
Here’s a basic example:
# debug_example.py (Debugging example)
def divide(x, y):
result = x / y
return result
# Run the debugger
import pdb; pdb.set_trace()
# Trigger the function with incorrect values
result = divide(10, 0)
print("Result:", result)
When the debugger is active, you can use commands like n
(next), c
(continue), and p
(print) to navigate through the code and inspect variables.
Testing and debugging are ongoing processes in software development. Regularly testing your code and using debugging tools will help you catch and resolve issues early in the development cycle.
In the next section, we’ll explore the deployment and scaling of your full-stack applications, bringing your work to a wider audience.
Deployment and Scaling
Congratulations on reaching the deployment and scaling phase of your full-stack web development journey!
Deployment involves making your application accessible to users, while scaling ensures it can handle increased load and traffic.
Let’s explore the key aspects of deploying and scaling Python web applications.
Deploying a Python Web Application
- Choose a Hosting Provider: Select a hosting provider that fits your needs. Popular choices include Heroku, AWS, Google Cloud, and DigitalOcean.
- Prepare Your Application: Ensure your application is ready for deployment. This involves configuring database connections, setting environment variables, and updating any absolute paths.
- Version Control: Use version control systems like Git to manage your code. Hosting platforms often integrate with Git for seamless deployment.
- Configure Web Server: Set up a web server to serve your application. Platforms like Heroku handle this automatically, but if you’re using a virtual server, consider options like Nginx or Apache.
- Environment Variables: Store sensitive information like database credentials as environment variables to enhance security.
- Static Files: If your application includes static files (CSS, images), configure the server to serve them efficiently. Django, for example, has a
collectstatic
management command. - Database Migration: Ensure your database schema is up-to-date. Most web frameworks provide migration tools to manage database changes.
- Domain Configuration: If you have a custom domain, configure it to point to your server’s IP address or domain provided by the hosting service.
Best Practices for Scaling
- Load Balancing: Distribute incoming traffic across multiple servers to prevent overload on a single server. Load balancers like HAProxy or services provided by cloud platforms can help.
- Caching: Implement caching strategies to reduce the load on your database and improve response times. Tools like Redis or Memcached can assist in caching.
- Horizontal Scaling: Increase capacity by adding more servers. Cloud platforms make it easy to scale horizontally by adding instances.
- Database Scaling: Choose a database that supports scaling. Consider sharding, replication, or using managed database services.
- Monitoring and Optimization: Implement monitoring tools to keep track of application performance. Identify bottlenecks and optimize code and database queries accordingly.
- Content Delivery Network (CDN): Utilize CDNs to cache and distribute static assets globally, reducing latency for users worldwide.
By following these deployment and scaling best practices, you’ll ensure that your full-stack web application is not only accessible to users but can also handle increased demand and traffic.
In the final section, we’ll explore advanced Python concepts, encouraging you to continue your learning journey and delve deeper into the world of full-stack development.
Advanced Concepts
As you embark on the final leg of your full-stack web development journey, let’s delve into advanced Python concepts that can elevate your skills to the next level.
While you’ve mastered the basics, these advanced topics will provide you with a deeper understanding of its capabilities.
Asynchronous Programming
Asynchronous programming allows your application to perform multiple tasks concurrently without waiting for each one to complete. This is crucial for handling tasks like network requests or database queries efficiently.
Python’s asyncio
module facilitates asynchronous programming.
Here’s a simple example using asyncio
:
import asyncio
async def async_task(name):
print(f"Start {name}")
await asyncio.sleep(2)
print(f"End {name}")
async def main():
tasks = [async_task("Task 1"), async_task("Task 2")]
await asyncio.gather(*tasks)
asyncio.run(main())
Decorators and Metaclasses
Decorators
Decorators in Python allow you to modify or extend the behavior of functions or methods. They are widely used for aspects like logging, authentication, and memoization.
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
Metaclasses
Metaclasses are classes for classes. They define the behavior of how classes are created. While not commonly used, they can be powerful in certain scenarios.
class MyMeta(type):
def __new__(cls, name, bases, dct):
dct['custom_attribute'] = 42
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=MyMeta):
pass
print(MyClass.custom_attribute)
Design Patterns in Python
Design patterns are reusable solutions to common problems in software design. Understanding and applying design patterns can lead to more maintainable and scalable code.
Example: Singleton Pattern
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance
# Usage
singleton1 = Singleton()
singleton2 = Singleton()
print(singleton1 is singleton2) # True
As you explore these advanced concepts, remember that they might not be necessary for every project.
However, having knowledge of them can provide elegant solutions to complex problems and showcase your expertise as a full-stack web developer.
Resources for Further Learning
Congratulations on completing this comprehensive guide to Python for full-stack web developers! As you continue your journey, it’s essential to stay curious and keep honing your skills.
Here are some valuable resources to further enhance your knowledge:
Recommended Books
- “Flask Web Development” by Miguel Grinberg: A comprehensive guide to building web applications using the Flask framework.
- “Django for Beginners” by William S. Vincent: A beginner-friendly introduction to web development with Django.
- “Effective Python: 90 Specific Ways to Write Better Python” by Brett Slatkin: Offers practical advice on writing clean, efficient, and Pythonic code.
Online Courses
- Coursera – “Python for Everybody” by University of Michigan: A specialization covering Python fundamentals and web development.
- Udemy – “Django for Beginners” by Navin Reddy: An in-depth course for beginners to master Django development.
- edX – “Introduction to Computer Science and Programming Using Python” by MIT: A foundational course covering Python programming principles.
Documentation and References
- Python Official Documentation: The official documentation is a treasure trove of information on Python features, libraries, and best practices.
- Django Documentation: The official Django documentation provides detailed guidance on using the Django web framework.
- Flask Documentation: The Flask documentation is an excellent resource for learning and mastering the Flask web framework.
Community Forums
- Stack Overflow – Python: A vibrant community where developers seek and share knowledge about Python.
- Django Forum: Connect with the Django community to ask questions, share insights, and learn from experienced developers.
- Reddit – r/learnpython: A supportive community for learners and experienced developers to discuss Python-related topics.
Networking Opportunities
- Meetup: Attend local or virtual meetups focused on Python and web development. It’s a great way to network with professionals in your area.
- LinkedIn: Join Python and web development groups on LinkedIn to connect with professionals, join discussions, and stay updated on industry trends.
Remember, the world of technology is ever-evolving, and continuous learning is key to staying ahead.
Stay curious, explore new technologies, and most importantly, enjoy the journey of becoming a skilled full-stack web developer.
Conclusion
As you wrap up this comprehensive beginner’s guide to Python for full-stack web developers, take a moment to reflect on your journey. You’ve gained a solid foundation in Python, explored backend and frontend development, mastered database interactions, and delved into advanced concepts.
Remember, becoming a proficient full-stack developer is an ongoing process. Embrace the mindset of continuous learning, as technology evolves, and new tools and frameworks emerge.
The skills you’ve acquired in this guide are the steppingstones to building innovative and scalable web applications.
Here are a few parting thoughts to carry with you:
- Stay Curious: Technology is a vast and ever-changing landscape. Stay curious and explore new technologies and methodologies.
- Practice Regularly: The more you code, the more you learn. Challenge yourself with projects, contribute to open source, and participate in coding exercises.
- Build a Portfolio: Showcase your skills by building a portfolio of projects. This not only demonstrates your capabilities to potential employers but also serves as a testament to your growth as a developer.
- Engage with the Community: Join developer forums, attend meetups, and engage with the broader developer community. Networking can lead to valuable insights, mentorship, and collaboration opportunities.
- Be Resilient: Learning to code and becoming a full-stack developer can be challenging, but perseverance is key. Embrace challenges as opportunities to learn and grow.
Keep in mind that every line of code you write contributes to your journey of becoming a proficient and creative developer. The skills you’ve acquired here set a strong foundation, and the possibilities are limitless.
Thank you for taking this learning journey with me. Whether you’re just starting or leveling up your skills, I wish you continued success in your full-stack web development endeavors.
Happy coding!
Leave a Reply