Welcome to the exciting world of frontend development—a crucial aspect of web development that brings websites to life, making them visually appealing and user-friendly.
Whether you’re just starting your journey into the realm of full-stack web development or looking to specialize in frontend, this beginner’s guide will provide you with a solid foundation.
What is Frontend Development?
Frontend development, also known as client-side development, involves crafting the user interface and user experience of a website.
In simpler terms, it’s what users see and interact with when they visit a site. From the layout and design to interactive features, frontend developers play a key role in ensuring a seamless and engaging user experience.
For example, think of a website like X (formerly Twitter). The timeline layout, the ability to tweet, and the interactive nature of the platform—all these elements are the result of frontend development.
Importance of Frontend Development in Web Development
The frontend is the face of a website, and first impressions matter. A well-designed and functional frontend not only attracts users but also keeps them engaged. It’s the difference between a website that users enjoy navigating and one that leaves them frustrated.
Consider the homepage of an e-commerce site. The layout, navigation menu, and product displays are all part of frontend development.
A well-crafted frontend can enhance the overall user experience, leading to increased user satisfaction and, ultimately, better business outcomes.
Fundamentals of Frontend Development
Frontend development encompasses the creation of the user interface and experience in web development. It revolves around fundamental concepts such as HTML, the structural language for web content; CSS, responsible for styling and design; and JavaScript, a programming language that introduces interactivity and dynamic content.
HTML
HTML, or HyperText Markup Language, is the backbone of web content. It provides the structure for web pages, using elements like headings, paragraphs, and lists to organize information.
For example, the following HTML snippet creates a simple webpage structure:
<!DOCTYPE html>
<html>
<head>
<title>My First Webpage</title>
</head>
<body>
<h1>Hello, World!</h1>
<p>This is my first webpage.</p>
</body>
</html>
Here, <html>
, <head>
, and <body>
are HTML elements defining the document structure.
Basic Structure of HTML Document
<html>
: The root element of an HTML page.<head>
: Contains meta-information about the document, such as the title.<title>
: Sets the title displayed on the browser tab.<body>
: The content of the webpage, including text, images, and other elements.
CSS
Cascading Style Sheets (CSS) complement HTML by styling and formatting the content. It allows developers to control layout, colors, and fonts.
Here’s an example:
body {
font-family: 'Arial', sans-serif;
background-color: #f4f4f4;
}
h1 {
color: #333;
}
p {
font-size: 16px;
}
This CSS code sets the background color of the page, changes the font for the body, adjusts the color of headings, and sets the font size for paragraphs.
Styling HTML Elements
- Selectors (e.g.,
body
,h1
,p
) target specific HTML elements. - Properties (e.g.,
color
,font-size
) define the style aspects. - Values (e.g.,
#333
,16px
) specify the characteristics.
Responsive Design Principles
CSS enables responsive design, ensuring websites look good on various devices. Media queries, like the one below, can adjust styles based on screen width:
@media only screen and (max-width: 600px) {
body {
font-size: 14px;
}
}
This query reduces the font size when the screen width is 600 pixels or less.
JavaScript
JavaScript adds interactivity and dynamic behavior to web pages. It’s a scripting language executed in the browser. For instance, the following script changes the content of an HTML element:
function changeText() {
document.getElementById('demo').innerHTML = 'New Content!';
}
This JavaScript function, when called, alters the content inside an HTML element with the id ‘demo’.
Basic Syntax and Data Types
JavaScript uses variables to store data. For example:
let number = 42;
let text = 'Hello, World!';
Here, number
holds a numeric value, and text
stores a string.
Manipulating the DOM
The Document Object Model (DOM) represents the structure of a document. JavaScript can manipulate it, enabling dynamic updates. For instance, the script below changes the background color when a button is clicked:
document.getElementById('myButton').addEventListener('click', function() {
document.body.style.backgroundColor = 'lightblue';
});
Here, the addEventListener
function listens for a click on the button with the id ‘myButton’ and changes the background color accordingly.
Event Handling
JavaScript responds to user actions through events. For example, a button click or a keypress triggers event handlers. The following HTML and JavaScript demonstrate a simple button click event:
<button onclick="alert('Button Clicked!')">Click me</button>
When the button is clicked, an alert pops up with the message ‘Button Clicked!’.
Frontend Frameworks & Libraries
Frontend frameworks are pre-written, standardized code structures that simplify the development of web applications. They provide a foundation, allowing developers to focus on building features rather than starting from scratch.
Here are some widely used frontend frameworks and their brief descriptions:
- React: Developed by Facebook, React is a JavaScript library for building user interfaces. It’s known for its component-based architecture, enabling the creation of reusable UI elements.
- Angular: Maintained by Google, Angular is a robust framework for building dynamic, single-page web applications. It follows the Model-View-Controller (MVC) architecture and offers a comprehensive set of tools for developers.
- Vue: Vue.js is a progressive JavaScript framework that is approachable and versatile. It provides reactive data binding and component-based architecture, making it easy to integrate into existing projects.
- Ember.js: Ember.js is a JavaScript framework that follows the convention over configuration (CoC) principle. It provides a structured way to build ambitious web applications with less boilerplate code.
- jQuery: jQuery is a fast and lightweight JavaScript library that simplifies HTML document traversal and manipulation, event handling, and animation. It’s particularly useful for handling cross-browser compatibility issues.
- Semantic-UI: Semantic-UI is a user interface framework that uses human-friendly HTML to create responsive and customizable designs. It aims to make web development faster and more intuitive.
- Backbone.js: Backbone.js is a lightweight JavaScript framework that provides structure to web applications. It offers models, views, collections, and routers to streamline development.
- Preact: Preact is a fast and lightweight alternative to React. It provides a similar API to React but with a smaller footprint, making it suitable for performance-critical applications.
- Svelte: Svelte is a component-based framework that shifts the work from the browser to the build step, resulting in smaller and faster runtime code. It’s known for its simplicity and efficiency.
- Foundation: Foundation is a responsive front-end framework that facilitates the rapid development of mobile-ready websites. It includes a flexible grid system and a variety of UI components.
CSS Preprocessors
CSS preprocessors, such as Sass and Less, extend the capabilities of traditional CSS. They introduce variables, nesting, and functions, enhancing code maintainability and organization.
Sass Example:
$primary-color: #3498db;
body {
background-color: $primary-color;
}
Here, $primary-color
is a variable holding the color value, promoting consistency and ease of updates.
Benefits and Integration with Frontend Development
- Variables: Define reusable values for consistent styling.
- Nesting: Organize styles hierarchically for improved readability.
- Functions: Create modular and reusable code snippets.
Expanding your knowledge beyond the foundational frameworks and exploring these additional tools can provide you with a diverse skill set as a frontend developer.
Responsive Web Design
Ensuring your web applications look and function seamlessly across various screen sizes is paramount. This is where responsive web design comes into play.
Responsive design is not just a trend; it’s a necessity in providing a positive user experience across desktops, tablets, and smartphones.
Consider a scenario where a user accesses your website on a desktop computer, and later, on a mobile device. Responsive design ensures that the content adapts and remains user-friendly regardless of the screen size, preventing the need for users to zoom in or scroll excessively.
Media Queries and Flexible Grid Layouts
Media Queries: Media queries are CSS rules that apply styles based on the characteristics of the device, such as screen width, height, or resolution. They allow developers to create breakpoints where styles can be adjusted for optimal viewing.
@media only screen and (max-width: 600px) {
/* Styles for screens up to 600px wide */
body {
font-size: 14px;
}
}
In this example, when the screen width is 600 pixels or less, the font size of the body is reduced for better readability on smaller screens.
Flexible Grid Layouts: A responsive design often involves using a flexible grid system that adjusts the layout based on the screen size. This ensures that the content is displayed in a visually pleasing and organized manner.
.container {
width: 100%;
max-width: 1200px;
margin: 0 auto;
}
.column {
width: 100%;
box-sizing: border-box;
padding: 10px;
}
@media only screen and (min-width: 768px) {
.column {
width: 50%;
}
}
Here, the container is set to 100% width, but with a maximum width of 1200px. The columns then adjust their width based on the screen size, using a 50% width when the screen is 768 pixels or wider.
Testing and Debugging for Different Devices
- Device Emulation Testing your web application on various devices can be done using browser developer tools that offer device emulation features. This allows you to simulate how your site will appear and function on different devices without the need for physical devices.
- Cross-Browser Compatibility In addition to various devices, it’s crucial to ensure cross-browser compatibility. Different browsers may interpret CSS and JavaScript differently, so testing on popular browsers like Chrome, Firefox, Safari, and Edge is essential.
Responsive web design not only caters to the current diverse device landscape but also prepares your application for future devices and screen sizes.
Browser Developer Tools
Browser Developer Tools are essential resources that empower frontend developers to inspect, debug, and optimize their code directly within web browsers. Understanding how to leverage these tools is fundamental for creating efficient and high-performing web applications.
Elements Panel: The Elements panel allows developers to inspect and manipulate the HTML and CSS of a page. You can view the document object model (DOM), modify styles, and debug layout issues.

Console Panel: The Console panel is a command-line interface where developers can execute JavaScript, log messages, and debug code. It’s a powerful tool for testing and troubleshooting.
console.log('Hello, Developer!');
Sources Panel: The Sources panel provides a full-featured code editor for debugging JavaScript. Developers can set breakpoints, step through code, and examine variables to identify and fix issues.
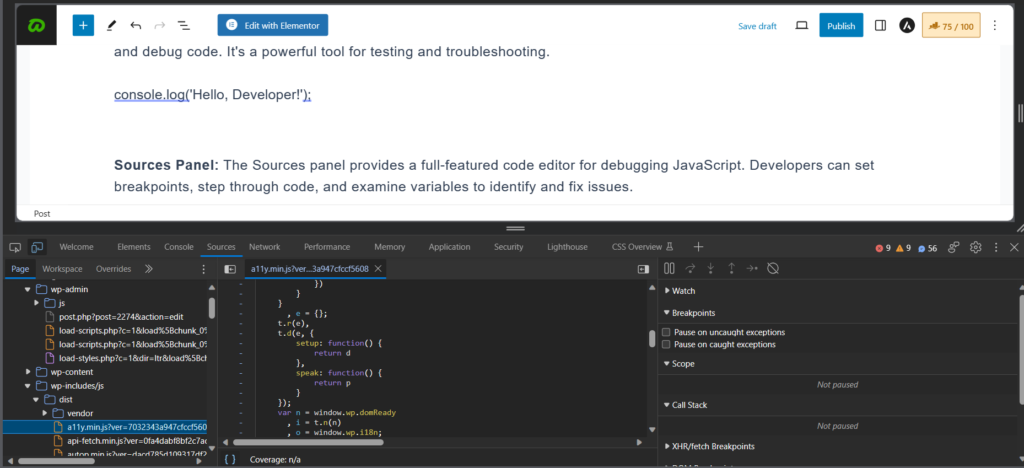
Using Developer Tools for Frontend Development
Browser Developer Tools are invaluable in streamlining the development and debugging process.
- Inspecting and Modifying Elements
- Right-click on an element and select “Inspect” to view and modify its HTML and CSS.
- Toggle device mode to emulate different devices and test responsive design.
- Debugging JavaScript
- Use the Console panel to log messages and execute JavaScript commands.
- Set breakpoints in the Sources panel to pause execution and analyze code step by step.
- Network Panel for Performance Analysis
- Monitor network requests to identify bottlenecks and optimize loading times.
- Analyze resource sizes, loading order, and response times.
Debugging and Optimizing Code
- Identifying and Fixing Issues
- Use the Console panel to catch errors and log information for debugging.
- Inspect elements to identify layout and styling issues.
- Performance Audits
- Leverage built-in performance audits to identify opportunities for optimization.
- Address issues such as unused CSS, large images, and inefficient JavaScript.
- Memory Profiling
- Detect and resolve memory leaks by using the Memory panel to profile JavaScript memory usage.
- Take heap snapshots to analyze memory consumption over time.
As you advance in your frontend development journey, mastering these tools will enhance your ability to create robust and efficient web applications.
Basics of Git Version Control
Version control is a crucial aspect of collaborative web development, enabling multiple developers to work on a project simultaneously without conflicts.
Git, a distributed version control system, is widely adopted in the development community. Understanding the basics of Git is essential for effective collaboration and code management.
1. Initializing a Git Repository: To start tracking changes in a project, initialize a Git repository using the following commands:
git init
This creates a hidden .git
directory to store version control information.
2. Committing Changes: After making modifications to your code, use the following commands to commit changes to the repository:
git add .
git commit -m "Commit message"
The git add .
command stages changes, and git commit
records them with a descriptive message.
Collaborative Development and Code Repositories
1. Remote Repositories: Git facilitates collaboration by allowing developers to work on a local copy of a repository and sync changes with a remote repository. Common remote hosting platforms include GitHub, GitLab, and Bitbucket.
git remote add origin <repository-url>
git push -u origin master
Here, origin
refers to the remote repository, and git push
updates the remote repository with local changes.
2. Branching and Merging: Git enables developers to create branches for feature development or bug fixes. Merging branches combines changes into the main branch.
git branch feature-branch
git checkout feature-branch
After making changes in the feature branch, merge it back into the main branch:
git checkout master
git merge feature-branch
Importance of Version Control in Full-Stack Development
- Code History and Rollbacks: Git preserves a detailed history of changes, making it easy to roll back to a previous state if issues arise. This ensures code stability and provides a safety net for experimentation.
- Collaborative Workflow: Version control allows multiple developers to work on different aspects of a project simultaneously. Changes can be integrated seamlessly, preventing conflicts and ensuring a smooth collaborative workflow.
- Code Review and Collaboration: Git repositories often integrate with tools that facilitate code reviews. Developers can comment on changes, suggest improvements, and ensure code quality before merging.
Version control with Git is a foundational skill for full-stack web developers. Whether you’re working on personal projects or contributing to a team, understanding Git’s principles enhances collaboration and code management.
Build Tools and Task Runners
Build tools and task runners automate repetitive tasks, optimize code, and enhance the development workflow. One prominent build tool is Webpack, widely used in modern web development for bundling and managing assets.
What is Webpack?
Webpack is a module bundler that takes disparate parts of your code, such as JavaScript, CSS, and images, and bundles them together in a way that’s optimized for the web. It also enables the use of modules and dependencies in the frontend.
npm install webpack webpack-cli --save-dev
Install Webpack using npm, and configure it through a webpack.config.js
file.
Key Features of Webpack
- Module Bundling: Combines modules into a single file, reducing the number of HTTP requests.
- Code Splitting: Divides code into smaller chunks, improving initial load times.
- Loaders: Processes different file types, such as transforming ES6 JavaScript to ES5.
- Plugins: Perform additional tasks, like minification or environment-specific configurations.
Automating Tasks with Task Runners (e.g., Gulp, Grunt)
Task runners automate repetitive tasks during development, such as minifying CSS, transpiling code, or optimizing images. Gulp and Grunt are popular task runners that simplify complex workflows.
npm install gulp-cli gulp --save-dev
Install Gulp using npm, and create a gulpfile.js
to define tasks.
Gulp vs. Grunt: A Brief Comparison
- Gulp: Emphasizes code over configuration, utilizing JavaScript for task definitions. Known for its streamlined and efficient API.
- Grunt: Configuration-driven, relying on JSON for task configurations. Offers a wide range of plugins and a more declarative approach.
Common Tasks with Gulp
- Minification: Reduce file sizes for improved load times.
- Concatenation: Combine multiple files into one for fewer HTTP requests.
- Sass Compilation: Process Sass/SCSS files into CSS.
- Live Reloading: Automatically refresh the browser when files change.
Both build tools and task runners play a pivotal role in optimizing code and enhancing development efficiency. Integrating these tools into your workflow can significantly improve the performance and maintainability of your web applications.
Web Performance Optimization
Web performance optimization is crucial for delivering a seamless user experience, improving page load times, and positively impacting search engine rankings. Users expect fast and responsive web applications, and optimizing performance contributes to user satisfaction and retention.
- Minification and Compression of Assets
- Minification: Removes unnecessary characters and spaces from code, reducing file sizes.
// Before Minification
function addNumbers(a, b) {
return a + b;
}
// After Minification
function addNumbers(a,b){return a+b;}
- Compression: Gzip and Brotli compression techniques reduce the size of assets sent to the browser, improving load times.
Caching Strategies
- Browser Caching: Utilize HTTP caching headers to instruct browsers to store static assets locally. This reduces the need to re-download resources on subsequent visits.
- Content Delivery Network (CDN): Distribute assets across multiple servers globally, ensuring faster delivery by serving content from the nearest server.
Lazy Loading of Images and Resources
- Lazy Loading: Load images and resources only when they come into the user’s viewport, reducing initial page load times.
<!-- Before Lazy Loading -->
<img src="image.jpg" alt="Example Image">
<!-- After Lazy Loading -->
<img src="placeholder.jpg" data-src="image.jpg" alt="Example Image" loading="lazy">
Async and Defer Attributes for Script Loading
- Async: Downloads and executes scripts asynchronously, allowing them to run independently without blocking other processes.
- Defer: Loads scripts in the background while parsing the HTML, then executes them in order after HTML parsing is complete.
<!-- Async Script Loading -->
<script async src="script.js"></script>
<!-- Defer Script Loading -->
<script defer src="script.js"></script>
Critical Rendering Path Optimization
- Above-the-Fold Content: Prioritize loading resources critical for the initial view of the page to enhance perceived performance.
- CSS and JavaScript Minification: Reduce file sizes and improve loading speed.
Web performance optimization is an ongoing process that involves continuous monitoring, analysis, and adjustment. By implementing these strategies, you can create web applications that not only meet user expectations but also perform efficiently across various devices and network conditions.
Accessibility in Frontend Development
Web accessibility ensures that websites and web applications are usable by everyone, regardless of disabilities or impairments. Incorporating accessibility best practices into frontend development is not only ethical but also crucial for reaching a diverse audience and providing an inclusive user experience.
- Semantic HTML
- Use semantic HTML elements to provide structure and meaning to content. For example, use
<nav>
for navigation,<article>
for articles, and<button>
for interactive elements.
- Use semantic HTML elements to provide structure and meaning to content. For example, use
- Proper Use of Headings and Labels
- Organize content with heading tags (
<h1>
to<h6>
) to create a logical hierarchy. - Associate form elements with labels to enhance screen reader compatibility.
- Organize content with heading tags (
<label for="username">Username:</label>
<input type="text" id="username" name="username">
- Keyboard Accessibility
- Ensure all interactive elements are navigable and operable using a keyboard. Test your website’s functionality without a mouse to identify potential issues.
- Implement focus styles to indicate the currently focused element.
- Contrast and Color Accessibility
- Maintain sufficient color contrast between text and background to improve readability, especially for users with visual impairments.
- Provide alternative cues for conveying information, avoiding reliance solely on color.
/* Example of Ensuring Sufficient Contrast */
body {
background-color: #ffffff;
color: #333333;
}
- Accessible Rich Internet Applications (ARIA)
- Use ARIA attributes to enhance the accessibility of dynamic content and interactions.
- ARIA roles, states, and properties provide additional information to assistive technologies.
<div role="alert" aria-live="assertive">
This is an important alert message.
</div>
Implementing Accessibility Best Practices
- Testing with Accessibility Tools
- Utilize browser extensions and online tools to assess the accessibility of your web application.
- Tools like Lighthouse, Axe, and Wave can identify potential issues and offer suggestions for improvement.
- User Testing with Diverse Audiences
- Conduct user testing with individuals representing diverse backgrounds and abilities.
- Collect feedback and adapt your design and development based on user experiences.
- Keeping Up with Web Content Accessibility Guidelines (WCAG)
- Familiarize yourself with the WCAG guidelines, a set of recommendations for creating accessible web content.
- Stay updated on the latest WCAG versions and integrate best practices into your development process.
By prioritizing accessibility in frontend development, you contribute to building a web environment that is inclusive and welcoming to all users.
Continuous Learning and Resources for Full-Stack Web Developers
The field of full-stack web development is dynamic and ever-evolving. Embracing a growth mindset is essential for staying relevant and mastering new technologies. Here are some key principles to foster continuous learning:
- Curiosity and Exploration
- Stay curious and explore emerging technologies, frameworks, and tools.
- Engage with online communities, forums, and developer events to stay informed about industry trends.
- Building Real-world Projects
- Apply your skills by working on real-world projects. This hands-on experience enhances problem-solving abilities and reinforces theoretical knowledge.
- Networking and Collaboration
- Connect with fellow developers, attend meetups, and participate in online forums.
- Collaborate on open-source projects to gain exposure to diverse perspectives and practices.
Resources for Full-Stack Web Developers
- Online Learning Platforms
- Coursera: Offers courses and specializations in web development, including full-stack development.
- Udacity: Provides nanodegree programs covering various aspects of full-stack development.
- edX: Features courses from universities and institutions worldwide, covering frontend and backend technologies.
- Documentation and Tutorials
- MDN Web Docs: Mozilla’s developer documentation is an excellent resource for web technologies, including HTML, CSS, and JavaScript.
- W3Schools: Offers tutorials and references for web development technologies and languages.
- Books and Publications
- “Eloquent JavaScript” by Marijn Haverbeke: A comprehensive guide to JavaScript and programming concepts.
- “You Don’t Know JS” series by Kyle Simpson: Explores JavaScript in-depth, focusing on essential concepts.
- Community and Forums
- Stack Overflow: A community-driven platform for asking and answering programming questions.
- GitHub Discussions: Engage with developers and communities associated with various projects on GitHub.
- Podcasts and Webinars
- Syntax Podcast: A podcast focused on web development, covering both frontend and backend topics.
- Google Developers YouTube Channel: Access webinars and talks on a variety of web development subjects.
Remember, the journey of a full-stack web developer is a continuous learning experience. Stay curious, be open to new challenges, and leverage the vast array of resources available to enhance your skills and contribute meaningfully to the world of web development.
Conclusion
Starting on the journey of becoming a full-stack web developer is both challenging and rewarding. Throughout this guide, we’ve covered the foundational concepts, tools, and practices that form the bedrock of your skill set. Let’s recap some key takeaways:
- Frontend Development Fundamentals
- Master HTML, CSS, and JavaScript as the core building blocks of web development.
- Understand the Document Object Model (DOM) and how it facilitates dynamic content manipulation.
- Frameworks and Libraries
- Explore popular frontend frameworks such as React, Angular, and Vue for efficient and scalable application development.
- Consider using CSS preprocessors like Sass and Less to enhance styling capabilities.
- Browser Developer Tools
- Learn to leverage browser developer tools for efficient debugging and optimization of your code.
- Explore the Elements, Console, and Sources panels for comprehensive insights into your web applications.
- Version Control with Git
- Grasp the essentials of Git for effective collaboration, versioning, and code management.
- Understand branching and merging strategies to streamline development workflows.
- Build Tools and Task Runners
- Integrate build tools like Webpack to bundle and optimize your assets for production.
- Explore task runners like Gulp and Grunt for automating repetitive tasks in your development workflow.
- Web Performance Optimization
- Prioritize performance optimization techniques, including asset minification, caching, and lazy loading.
- Implement practices that enhance the critical rendering path and improve user experience.
- Accessibility in Frontend Development
- Embrace semantic HTML, proper labeling, and keyboard accessibility for an inclusive user experience.
- Test and validate your website’s accessibility using tools and user testing with diverse audiences.
- Continuous Learning and Resources
- Cultivate a growth mindset and stay curious about emerging technologies in the dynamic field of web development.
- Utilize online learning platforms, documentation, books, and community engagement to continually enhance your skills.
As you continue your full-stack web development journey, remember that learning is an ongoing process. The technology landscape evolves, and staying adaptable and open to new challenges is key to your success.
Keep coding, building, and contributing to the vibrant community of web developers. Best of luck on your exciting adventure in the world of full-stack web development!
Leave a Reply