Collaboration lies at the heart of successful full-stack development, and mastering tools that facilitate teamwork is crucial.
GitHub, a robust version control platform, stands out as an essential companion for aspiring full-stack web developers.
In this guide, we will explore GitHub, providing you with the foundational knowledge needed to become a collaborative coding maestro.
Quick Links
The Significance of Collaboration in Full-Stack Development
In the dynamic landscape of web development, projects often involve multiple contributors working simultaneously.
Whether you’re collaborating with team members, contributing to open-source projects, or simply managing your own codebase, effective collaboration streamlines the development process.
GitHub serves as a centralized hub where developers unite, contributing their expertise to create seamless and powerful web applications.
Revealing GitHub’s Power
GitHub is not just a platform; it’s a powerhouse that empowers developers to manage and track changes to their code effortlessly.
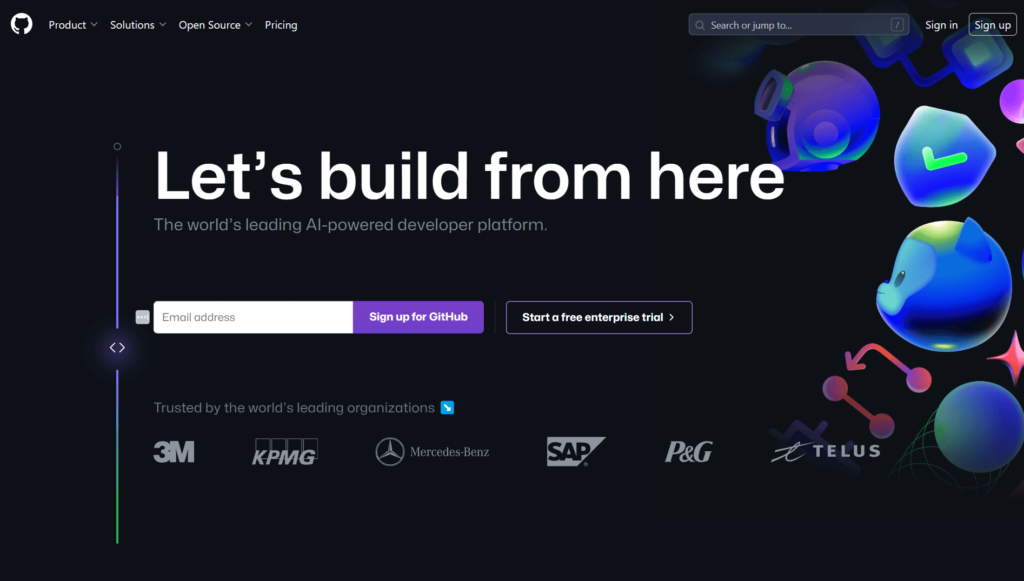
Think of it as a digital space where your code lives, breathes, and evolves. As we delve into this guide, you’ll discover how to harness GitHub’s capabilities to enhance your collaborative coding journey.
Now, let’s roll up our sleeves and get started with the basics: setting up your GitHub account.
# Example command for setting up a Git configuration
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Setting Up Your GitHub Account
Creating Your GitHub Account
The first step on your GitHub journey is to create an account. Head to GitHub’s Signup Page and follow the straightforward registration process.
Choose a professional username and provide an email address to ensure a seamless experience as you venture into collaborative coding.
Crafting Your Professional Profile
Once registered, invest time in customizing your GitHub profile. Your profile is your digital identity in the coding world.
Add a profile picture, a concise bio, and links to your portfolio or personal website.
This not only enhances your professional image but also makes it easier for potential collaborators or employers to learn more about your skills and contributions.
Now, let’s dive into the core of GitHub—understanding repositories.
# Example command for cloning a repository
git clone https://github.com/example/repository.git
Understanding Repositories
Definition and Purpose of Repositories
In GitHub, a repository, or “repo” for short, is a centralized space where your project lives. It contains all the files, folders, and assets related to your application.
The repository keeps track of changes, making it easy to collaborate with others without stepping on each other’s toes.
Think of a repository as a virtual workspace where developers gather to contribute, modify, and enhance the project.
Each repository has its unique URL, allowing collaborators to access and clone it to their local machines.
Creating Your First Repository
To create a repository, navigate to your GitHub profile, and click on the “+ New” button. Fill in the repository name, add a brief description, and choose whether it should be public or private. Initializing the repository with a README is a good practice, as it provides a starting point for your project.
Once your repository is created, you’ll be greeted with a welcoming screen, signaling the birth of your collaborative coding space. Now, let’s explore the next steps—cloning and forking repositories.
# Example command for creating a new repository
echo "# My Project" >> README.md
git init
git add README.md
git commit -m "Initial commit"
git branch -M main
git remote add origin https://github.com/your-username/your-repository.git
git push -u origin main
Cloning and Forking
Understanding Cloning and Forking on GitHub
Cloning and forking are fundamental actions on GitHub that allow you to bring a repository from the platform to your local development environment.
Let’s break down these concepts:
- Cloning: Cloning is like making a copy of a repository onto your local machine. This enables you to work on the project, make changes, and experiment without affecting the original repository on GitHub.
- Forking: Forking, on the other hand, creates a personal copy of someone else’s repository under your GitHub account. It’s a way of saying, “I want to contribute to this project.” You can make changes in your forked repository and then propose these changes back to the original repository through pull requests.
Hands-On Guide to Cloning and Forking
Cloning a Repository:
To clone a repository, navigate to the repository’s main page on GitHub, click the “Code” button, and copy the provided URL. Open your terminal and use the following commands:
git clone https://github.com/example/repository.git
Now, you have a local copy of the repository on your machine.
Forking a Repository:
- On GitHub, navigate to the repository you want to fork.
- Click the “Fork” button in the top-right corner of the page.
- Select your account as the destination for the fork.
Once forked, you can clone the repository to your local machine using the previous cloning commands.
Now that you have a local copy of a repository let’s explore branching strategies in the context of GitHub.
# Example command for forking a repository
gh repo fork example/repository
Branching Strategies
Importance of Branching in Collaborative Development
Branching is a pivotal concept in version control, allowing developers to work on different features or bug fixes without disrupting the main codebase.
In GitHub, branches act as isolated environments where you can introduce changes independently and merge them back seamlessly.
Main Branches:
- Main/Branch: This is often the default branch where the stable and production-ready code resides.
- Feature Branches: These branches are created for specific features or enhancements. They allow developers to work on new functionalities without affecting the main codebase.
- Bug Fix Branches: Created to address and fix issues in the code, bug fix branches isolate problem-solving efforts.
Overview of Common Branching Strategies
Feature Branch Workflow:
- Create a Feature Branch:
git checkout -b feature/new-feature main
- Make Changes and Commit:
git add .
git commit -m "Implemented new feature"
- Merge Changes:
git checkout main
git merge feature/new-feature
2. Gitflow Workflow:
Gitflow is a branching model that defines a strict branching structure. It includes:
- Master Branch: Stable code for production.
- Develop Branch: Ongoing development branch.
- Feature Branches: For developing new features.
- Release Branches: Prepare for a new release.
Example Gitflow Commands:
- Start a New Feature:
git flow feature start new-feature
- Finish a Feature:
git flow feature finish new-feature
Understanding and adopting an appropriate branching strategy streamlines collaboration and keeps your codebase organized.
Next, we’ll explore the art of committing changes in GitHub.
# Example command for creating a new feature branch
git branch feature/new-feature
git checkout feature/new-feature
Committing Changes
Understanding Commits and Their Role in Version Control
Commits in GitHub serve as snapshots of your project at a specific point in time. Each commit represents a set of changes made to the codebase.
This granular approach allows you to track the evolution of your project and revert to previous states if needed.
Anatomy of a Commit:
- Unique Identifier (SHA): A long alphanumeric code uniquely identifying the commit.
- Author and Timestamp: Information about who made the commit and when.
- Commit Message: A concise and informative message describing the changes made.
Best Practices for Writing Clear Commit Messages
Writing clear commit messages is crucial for effective collaboration and code maintenance. Follow these best practices:
- Be Descriptive: Clearly describe the purpose of the commit in the message.
- Use Present Tense: Write commit messages in the present tense to convey ongoing actions.
- Keep it Concise: Be brief while ensuring the message conveys the necessary information.
Now, let’s dive into committing changes with an example:
# Stage changes for commit
git add .
# Commit changes with a descriptive message
git commit -m "Implemented user authentication feature"
As you continue your journey in collaborative coding, mastering the art of writing meaningful commit messages will enhance the clarity and understanding of your project’s development history.
Next up, we’ll explore the world of pull requests in GitHub.
# Example command for staging and committing changes
git add .
git commit -m "Implemented user authentication feature"
Pull Requests
Understanding the Concept of Pull Requests
Pull requests (PRs) are a cornerstone of collaboration on GitHub. They provide a platform for proposing changes, discussing modifications, and eventually merging code into the main branch.
PRs facilitate a transparent and organized workflow, making it easy for contributors to collaborate effectively.
Key Components of a Pull Request:
- Title and Description: Clearly articulate the purpose of the pull request.
- Commits: View the individual commits included in the pull request.
- Changes: A visual representation of the code modifications introduced.
Step-by-Step Guide on Creating and Reviewing Pull Requests
Creating a Pull Request:
- Navigate to the Repository: Go to the main page of the repository on GitHub.
- Create a New Branch: If you’re working on a new feature, create a dedicated branch for it.
- Make Changes and Commit: Implement the desired changes and commit them to your branch.
- Initiate a Pull Request:
- On the repository page, click on the “Pull Requests” tab.
- Click the “New Pull Request” button.
- Compare Changes: Select the branches you want to compare (usually your feature branch against the main branch).
- Create Pull Request:
- Write a descriptive title and provide details about the changes.
- Click “Create Pull Request” to open the PR.
Reviewing a Pull Request:
- Access the Pull Request: Go to the “Pull Requests” tab on the repository page.
- Review Changes: Examine the code modifications, comments, and discussions.
- Provide Feedback: Comment on specific lines of code to discuss improvements or ask questions.
- Approve or Request Changes: Approve the pull request if everything looks good, or request changes if modifications are needed.
- Merge the Pull Request: Once approved, the pull request can be merged into the main branch.
Mastering the pull request workflow enhances collaboration by fostering effective communication and code review.
As you progress, you’ll find pull requests to be an integral part of the collaborative coding experience. Next, let’s explore different collaborative workflows on GitHub.
# Example command for creating a new branch
git checkout -b feature/new-feature main
Collaborative Workflows
Overview of Different Collaborative Workflows on GitHub
GitHub offers flexibility in choosing collaborative workflows based on the nature and scale of your project. Let’s explore two popular workflows:
Centralized Workflow:
- Single Main Branch: The project has a single main branch, often named “main” or “master.”
- Individual Feature Branches: Developers create feature branches for their work.
- Pull Requests: Changes are proposed and reviewed through pull requests before merging into the main branch.
Forking Workflow:
- Personal Forks: Each developer forks the main repository to their personal GitHub account.
- Feature Branches in Personal Forks: Developers create feature branches in their forks.
- Pull Requests to Main Repository: Changes are proposed through pull requests from personal forks to the main repository.
Choosing the Right Workflow for Your Full-Stack Projects
The choice between centralized and forking workflows depends on project requirements, team dynamics, and collaboration preferences. Consider the following factors:
- Team Size: Centralized workflows may suit smaller teams, while forking workflows scale well for larger collaborations.
- Project Scale: Forking workflows are advantageous for open-source projects with external contributors.
- Access Control: Centralized workflows offer more control over the main repository, while forking workflows allow contributors to maintain their own forks.
Selecting the appropriate workflow streamlines collaboration, ensuring a smooth and efficient development process.
Now, let’s delve into the world of issue tracking on GitHub.
# Example command for creating a new feature branch
git checkout -b feature/new-feature main
Issue Tracking
Introduction to GitHub Issues for Bug Tracking and Feature Requests
GitHub Issues serve as a dynamic tool for tracking bugs, discussing enhancements, and managing tasks within a project.
Whether you’re fixing a bug, adding a new feature, or improving existing functionality, issues provide a centralized space for collaboration and communication.
Key Features of GitHub Issues:
- Title and Description: Clearly describe the issue, including its purpose and context.
- Labels: Categorize issues with labels, such as bug, enhancement, or help wanted.
- Assignees: Designate individuals responsible for addressing specific issues.
Implementing Effective Issue Tracking in Your Projects
Creating an Issue:
- Navigate to the Issues Tab: Go to the “Issues” tab on the repository page.
- New Issue: Click the “New Issue” button.
- Describe the Issue: Write a detailed title and description of the issue.
- Labels and Assignees: Add relevant labels and assign the issue to the appropriate team member.
Managing and Resolving Issues:
- Issue Board: Utilize the issue board to get an overview of the project’s status.
- Discussion: Engage in discussions within the issue thread to gather feedback and collaborate on solutions.
- Closing Issues: Once an issue is resolved, close it with a concise comment summarizing the resolution.
Effective issue tracking ensures a systematic approach to project development, making it easier for contributors to identify, address, and collaborate on tasks.
As you integrate issue tracking into your workflow, you’ll find it to be an invaluable tool for maintaining project momentum.
Next, let’s explore best practices for maintaining a clean and organized GitHub repository.
GitHub Best Practices
Coding Conventions and Style Guides for Collaborative Projects
Maintaining consistency in your codebase is essential for collaborative projects.
Adopting coding conventions and following style guides ensures that the code is readable, understandable, and accessible to all contributors.
Coding Conventions:
- Indentation: Choose a consistent indentation style (spaces or tabs) and apply it uniformly throughout the project.
- Naming Conventions: Define clear and descriptive names for variables, functions, and classes.
- Comments: Use comments to explain complex sections of code, providing insights for collaborators.
Style Guides:
- JavaScript: Follow popular style guides like Airbnb or Google’s JavaScript Style Guide.
- Python: Adhere to the PEP 8 style guide for Python code.
- CSS: Embrace a consistent naming convention and formatting style for CSS rules.
Tips for Maintaining a Clean and Organized GitHub Repository
1. Clear Repository Structure:
- Organize your project with a clear directory structure, separating code, documentation, and assets.
2. README Documentation:
- Craft a comprehensive README.md file with project information, setup instructions, and usage guidelines.
3. Use Version Tags:
- Tag releases with version numbers to mark stable points in your project’s development.
4. Continuous Integration:
- Implement continuous integration tools to automate testing and ensure code quality.
5. Code Reviews:
- Regularly conduct code reviews to maintain code quality and catch potential issues early.
Adopting best practices not only enhances collaboration but also contributes to the long-term maintainability and scalability of your full-stack projects on GitHub.
As you embrace these practices, you’ll find that a well-organized repository fosters a positive collaborative environment.
Conclusion
GitHub is not just a platform for version control; it’s a hub where ideas flourish, problems are solved, and innovations take shape. The collaborative power of GitHub transforms individual efforts into a collective force driving the evolution of web applications.
As you progress in your full-stack development journey, continue exploring GitHub’s features, staying updated on best practices, and embracing the collaborative spirit of the developer community.
Whether you’re working on personal projects, contributing to open source, or collaborating with a team, GitHub will be your ally in creating exceptional web experiences.
Armed with the knowledge gained from this guide, you’re well on your way to becoming a proficient full-stack developer, adept at leveraging GitHub for seamless collaboration. Keep coding, keep collaborating, and enjoy the exciting journey ahead.
Happy coding!
Leave a Reply