Version control is a critical aspect of web development, providing a systematic way to manage and track changes in code.
It ensures collaboration among developers, safeguards against errors, and allows for the seamless evolution of projects.
Among the various version control systems, Git stands out as a robust and versatile tool that has become a cornerstone in the web development toolkit.
Quick Links
Brief overview
In web development, projects are in a constant state of evolution. Multiple developers often collaborate on the same codebase, making it crucial to have a system that organizes, tracks, and documents changes.
Version control serves this purpose, offering a structured approach to managing code modifications.
It not only facilitates collaboration but also provides a safety net, enabling developers to roll back to previous versions if issues arise.
Introduction to Git as a powerful version control system
Git, developed by Linus Torvalds, is a distributed version control system renowned for its efficiency and flexibility. Unlike centralized systems, every developer working with Git has their own local repository, allowing for autonomous work.
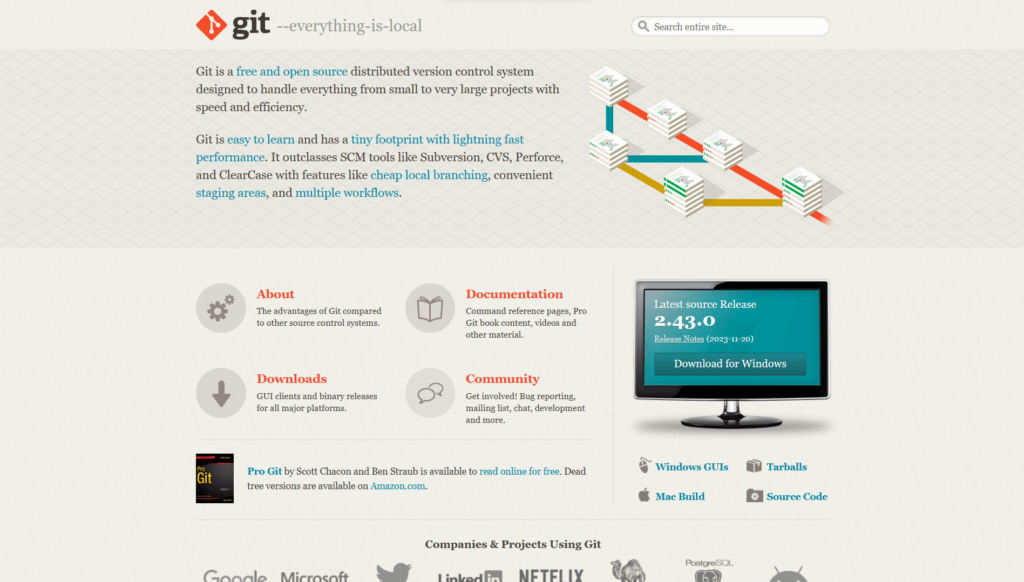
Changes made locally can later be merged with the main project. This decentralized nature enhances collaboration, speeds up development, and provides a reliable mechanism for tracking changes.
With its vast ecosystem and compatibility with platforms like GitHub and GitLab, Git has become the go-to choice for developers across the globe.
Its ability to handle both small-scale projects and large, complex codebases makes it an invaluable asset in the realm of web development.
In the following sections, we’ll delve into the practical aspects of using Git, from installation and configuration to advanced collaboration techniques.
Setting Up Git
Setting up Git is the initial step towards harnessing its power for version control in web development.
This section will guide you through the installation process on different operating systems and help you configure basic user information.
Installation process for Git on different operating systems
Installing Git is a straightforward process, but the steps may vary depending on your operating system.
- Windows:
- Visit the official Git website and download the installer.
- Run the installer and follow the on-screen instructions.
- Open the Git Bash terminal to start using Git commands.
- Mac:
- Mac users can install Git using Homebrew by running
brew install git
in the terminal. - Alternatively, download the installer from the official website and follow the installation instructions.
- Mac users can install Git using Homebrew by running
- Linux:
- For Debian-based systems, use
sudo apt-get install git
in the terminal. - Red Hat-based systems can use
sudo yum install git
.
- For Debian-based systems, use
Configuration of basic user information
Before diving into Git, it’s essential to configure your user information. This ensures that your commits are attributed correctly.
# Set your username
git config --global user.name "Your Name"
# Set your email address
git config --global user.email "your.email@example.com"
These global configurations apply to all your Git repositories. You’re now set up with Git on your machine and ready to initiate your first repository.
In the upcoming sections, we’ll explore the fundamental concepts of Git, such as creating repositories, branching, merging, and collaborating with remote repositories.
Creating Your First Repository
Now that Git is set up on your machine, let’s jump into creating your first repository. This involves initializing a new Git repository, understanding the staging area, and committing changes.
Initializing a new Git repository
To start version controlling a project, you need to initialize a Git repository. Navigate to your project directory in the terminal and run:
# Initialize a new Git repository
git init
This command creates a hidden .git
directory in your project, where Git stores its internal data.
Understanding the staging area and committing changes
Git uses a staging area to prepare changes before committing them. After making modifications to your files, use the following commands:
# Add changes to the staging area
git add .
# Commit changes with a meaningful message
git commit -m "Initial commit"
The git add
command stages your changes, and git commit
records them in the repository with a descriptive message. This process allows for a granular and organized approach to tracking modifications.
In the upcoming sections, we’ll delve into advanced Git concepts, such as branching and merging, to enhance your ability to manage and collaborate on projects effectively.
Branching and Merging
As your projects evolve, managing different aspects concurrently becomes crucial. This is where branching and merging in Git come into play.
Introduction to branches and their significance
In Git, a branch is a separate line of development, allowing you to work on features or bug fixes without affecting the main codebase.
The main branch, often named master
or main
, represents the stable version of your project.
# Create a new branch
git branch feature-branch
# Switch to the new branch
git checkout feature-branch
This example creates a new branch called feature-branch
and switches to it, providing a clean slate to implement new features.
Creating and switching between branches
To streamline the process, you can use the following shorthand command:
# Create and switch to a new branch
git checkout -b feature-branch
This command combines branch creation and switching in one step.
Merging branches to incorporate changes
Once you’ve completed work on a branch and are satisfied with the changes, it’s time to merge it back into the main branch.
# Switch to the main branch
git checkout main
# Merge the feature branch into the main branch
git merge feature-branch
Merging integrates the changes made in the feature branch into the main branch, ensuring a cohesive codebase.
In the following sections, we’ll explore remote repositories, resolving conflicts, Git best practices, and collaborative workflows.
These concepts will empower you to work seamlessly on projects with multiple developers.
Remote Repositories
This section covers linking local repositories to remote repositories (e.g., GitHub, GitLab) and pushing and pulling changes between them.
Linking local repositories to remote repositories
Before linking, create a repository on your preferred hosting platform (e.g., GitHub).
# Link your local repository to a remote repository
git remote add origin <remote-repository-url>
This associates your local repository with the remote one, enabling seamless collaboration.
Pushing and pulling changes between local and remote repositories
To share your local changes with the remote repository, use the following commands:
# Push changes to the remote repository
git push -u origin main
Conversely, to incorporate changes from the remote repository into your local workspace:
# Pull changes from the remote repository
git pull origin main
These commands ensure that your local and remote repositories stay in sync, allowing for efficient collaboration among team members.
In the upcoming sections, we’ll explore conflict resolution, Git best practices, and advanced collaboration techniques, empowering you to navigate the intricacies of web development seamlessly.
Resolving Conflicts
In the collaborative landscape of web development, conflicts can arise when multiple contributors modify the same part of a file concurrently. Resolving these conflicts is a crucial skill in maintaining code integrity.
Understanding merge conflicts and their causes
Merge conflicts occur when Git is unable to automatically merge changes due to conflicting modifications in different branches. Understanding the causes helps in preemptively addressing conflicts.
# Example of a conflict: conflicting changes in a file
<<<<<<< HEAD
Code from the current branch
=======
Code from the incoming branch
>>>>>>> incoming-branch
This conflict marker delineates the conflicting sections, with the changes from the current branch above =======
and those from the incoming branch below.
Strategies for resolving conflicts and maintaining code integrity
- Manual resolution:
- Review conflicting changes and manually choose which version to keep.
- Remove conflict markers and commit the resolved file.
- Merge tools:
- Use graphical or command-line merge tools to visualize and merge conflicting changes.
# Launch a merge tool
git mergetool
Conflicts are inevitable in collaborative projects, but adept conflict resolution ensures a cohesive and functional codebase.
In the following sections, we’ll explore Git best practices, including committing regularly, writing meaningful commit messages, using .gitignore
to ignore files and directories, and employing tags for versioning and releases.
Git Best Practices
Adhering to best practices enhances the efficiency and maintainability of your Git-managed projects.
This section covers committing regularly, writing meaningful commit messages, using .gitignore
, and employing tags for versioning and releases.
Committing regularly and writing meaningful commit messages
Frequent and well-documented commits provide a clear history of project changes. Aim for atomic commits that address specific features, bug fixes, or improvements.
# Commit changes with a descriptive message
git commit -m "Add feature: user authentication"
Meaningful commit messages make it easier to understand the evolution of the project and pinpoint specific changes.
Ignoring files and directories using .gitignore
Not all files belong in version control. Use a .gitignore
file to specify files and directories that Git should ignore.
# Example .gitignore file
node_modules/
*.log
This prevents irrelevant files, like dependencies or logs, from cluttering your repository.
Using tags for versioning and releases
Tags are references to specific points in Git history, commonly used for versioning and releases.
# Create a lightweight tag
git tag v1.0.0
# Push tags to the remote repository
git push origin --tags
Tags provide a snapshot of the project at a particular point, aiding in version management.
In the subsequent sections, we’ll delve into collaborative workflows, including pull requests and code reviews, fostering effective collaboration among multiple developers.
Collaboration with Git
Effective collaboration is at the heart of successful web development projects. This section explores collaborative workflows with multiple developers, encompassing pull requests and code reviews.
Collaborative workflows with multiple developers
Collaboration often involves multiple developers working on different features or bug fixes concurrently. A common approach is the feature branch workflow:
- Create a feature branch: Each developer creates a branch for their specific task or feature.
git checkout -b feature-branch
- Work independently: Developers work on their respective branches independently, avoiding conflicts during the development phase.
- Merge into the main branch: Once the feature is complete and tested, the developer merges their branch into the main branch.
git checkout main
git merge feature-branch
Pull requests and code reviews for effective collaboration
Pull requests (PRs) are a mechanism for proposing changes and initiating code reviews.
They facilitate discussion, feedback, and approval before merging into the main branch.
- Create a pull request: Push your feature branch to the remote repository and create a pull request.
git push origin feature-branch
- Code review: Team members review the code, provide feedback, and discuss potential improvements directly on the pull request.
- Merge after approval: Once the code is approved, the pull request can be merged into the main branch.
git merge feature-branch
This collaborative workflow ensures a systematic approach to incorporating changes, fostering a cohesive and well-maintained codebase.
In the concluding sections, we’ll recap key Git concepts for aspiring full-stack web developers and provide encouragement to practice and explore advanced Git features.
Conclusion
As we reach the culmination of this journey into Git, let’s recap key concepts that will empower aspiring full-stack web developers.
Recap of key Git concepts
- Version Control Importance: Understand the significance of version control in web development for collaboration, error tracking, and project evolution.
- Git as a Powerful Tool: Grasp the power of Git as a distributed version control system, offering efficiency, flexibility, and seamless collaboration.
- Setting Up Git: Learn the installation process on different operating systems and configure basic user information for a personalized Git experience.
- Creating Your First Repository: Initiate a Git repository, comprehend the staging area, and commit changes to establish a structured version control system.
- Branching and Merging: Explore the creation of branches, the significance of branch management, and the process of merging changes for a cohesive codebase.
- Remote Repositories: Link local repositories to remote platforms like GitHub, enabling efficient sharing and collaboration among developers.
- Resolving Conflicts: Gain proficiency in identifying and resolving merge conflicts, ensuring code integrity in collaborative projects.
- Git Best Practices: Embrace best practices, including regular commits, meaningful commit messages, effective use of
.gitignore
, and employing tags for versioning. - Collaboration with Git: Implement collaborative workflows, featuring feature branches, pull requests, and code reviews, to enhance teamwork and project organization.
Challenge yourself to explore advanced Git features, such as rebase, interactive staging, and custom workflows.
Continuous learning and hands-on experience will refine your Git skills and elevate your capabilities in web development.
Git is not just a tool; it’s a skill that grows with every project you undertake. Embrace the Git workflow, collaborate with passion, and let your coding journey flourish.
Happy coding!
Leave a Reply