As full-stack web developers, the choices we make in our technology stack can significantly impact the performance and scalability of our applications.
One often overlooked but powerful tool in our arsenal is Redis.
Redis isn’t just another database; it’s a high-performance, in-memory data store that brings a host of benefits to the table.
Whether you’re a seasoned developer or just starting on your coding journey, understanding Redis and its strategic use can elevate your web development game.
// Example of a simple Redis connection in Node.js
const redis = require('redis');
const client = redis.createClient();
client.on('connect', () => {
console.log('Connected to Redis Server');
});
client.on('error', (err) => {
console.error(`Redis Error: ${err}`);
});
In this article, we’ll delve into the world of Redis and explore seven compelling reasons why and when full-stack web developers should consider integrating it into their projects.
Quick Links
Brief Overview
What is Redis and Key Features
Redis, short for Remote Dictionary Server, is an advanced key-value store renowned for its exceptional speed and versatility.
Unlike traditional databases, it operates primarily in-memory, making data retrieval lightning-fast.
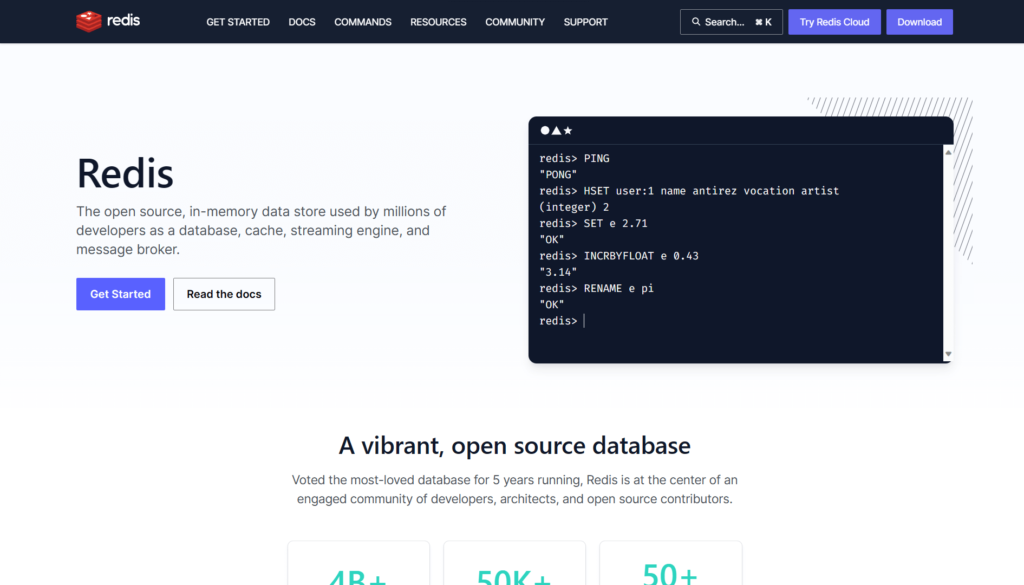
Its key features include support for various data structures such as strings, hashes, lists, sets, and more.
// Example of storing data in Redis as a key-value pair
client.set('user:1:name', 'John Doe', (err, reply) => {
if (err) {
console.error(`Error setting data: ${err}`);
} else {
console.log(`Data set successfully: ${reply}`);
}
});
Redis vs. Other Database Systems
Redis stands out in the crowded database landscape due to its in-memory nature and efficient data structures.
Unlike traditional relational databases, it excels in scenarios where quick data access and manipulation are crucial. While SQL databases are structured and query-heavy, its simplicity and speed make it a preferred choice for certain use cases.
In the upcoming sections, we’ll explore specific advantages that it brings to the table for full-stack web developers.
#1 – Speed and Performance
Redis In-Memory Storage and Its Impact on Speed
One of its standout features is its in-memory storage model. Unlike traditional databases that rely on disk storage, it keeps data in RAM, leading to unparalleled speed in data retrieval and storage.
This makes it an ideal choice for scenarios where low-latency and high-throughput are paramount.
// Example of retrieving data from Redis
client.get('user:1:name', (err, reply) => {
if (err) {
console.error(`Error retrieving data: ${err}`);
} else {
console.log(`Retrieved data: ${reply}`);
}
});
Real-World Examples of Performance Improvements
Consider a scenario where a full-stack application requires rapid retrieval of user authentication tokens.
By leveraging Redis to store these tokens in-memory, the application can achieve near-instantaneous token validation, enhancing user experience and overall application performance.
In the following sections, we’ll explore how its data structure flexibility further contributes to the efficiency of full-stack web development.
#2 – Data Structure Flexibility
Overview of Redis Data Structures
The versatility shines through its support for a variety of data structures. These include strings, hashes, lists, sets, and more.
Each data structure serves a unique purpose, empowering developers to model their data in a way that best suits the requirements of their application.
// Example of using a Redis hash to store user data
client.hmset('user:1', {
name: 'Jane Doe',
age: 28,
email: 'jane@example.com'
}, (err, reply) => {
if (err) {
console.error(`Error storing hash data: ${err}`);
} else {
console.log(`Hash data stored successfully: ${reply}`);
}
});
Benefits in Various Scenarios
Consider a scenario where an e-commerce application needs to track inventory. Its set data structure can efficiently handle this by storing unique product IDs.
The flexibility to choose the right data structure for the task at hand makes it a powerful ally for full-stack developers, allowing for streamlined and efficient data management.
In the upcoming sections, we’ll delve into how its scalability and real-time capabilities contribute to its appeal for full-stack web developers.
#3 – Scalability
Redis as a Scalable Solution for Handling Large Datasets
In full-stack web development, scalability is a critical factor, especially as applications grow and user bases expand.
Its architecture is inherently designed for scalability, making it an excellent choice for handling large datasets with ease.
// Example of using Redis for caching to enhance scalability
const cacheKey = 'product:catalog';
const cachedData = await client.get(cacheKey);
if (cachedData) {
// Use cached data
console.log('Data retrieved from cache:', cachedData);
} else {
// Fetch data from the database and store it in Redis for future use
const databaseData = await fetchDataFromDatabase();
client.set(cacheKey, JSON.stringify(databaseData));
console.log('Data retrieved from the database:', databaseData);
}
Examples of Seamless Scaling in Full-Stack Applications
Imagine a social media platform experiencing a surge in user activity. The application can seamlessly scale by distributing the workload across multiple instances.
Its ability to handle increasing loads without compromising performance ensures a smooth scaling experience for full-stack developers.
As we move forward, we’ll explore how its real-time capabilities play a pivotal role in enhancing user interactivity in full-stack applications.
#4 – Real-time Capabilities
Pub/Sub Mechanism for Real-time Communication
Real-time communication is a game-changer in today’s dynamic web applications, and it excels in this domain with its Publish/Subscribe (Pub/Sub) mechanism.
This feature allows different parts of your full-stack application to communicate instantly, opening the door to real-time updates and interactive user experiences.
// Example of using Pub/Sub for real-time notifications
const channel = 'notifications';
// Subscribe to the channel
client.subscribe(channel);
// Listen for incoming messages
client.on('message', (channel, message) => {
console.log(`Received message on channel ${channel}: ${message}`);
});
// Publish a message to the channel
client.publish(channel, 'New notification: You have a new message!');
Use Cases of Real-time Features in Full-Stack Web Development
Consider a chat application where users expect instant message delivery. Pub/Sub can be employed to notify connected clients of new messages in real-time, creating a seamless and engaging chat experience.
The ability to integrate real-time features with ease positions it as a valuable asset for full-stack developers aiming to enhance user interactivity.
In the upcoming sections, we’ll explore how it seamlessly integrates with caching strategies, offering performance improvements that can elevate your full-stack web applications.
#5 – Integration with Caching Strategies
Redis as a Caching Solution for Improved Performance
Caching is a proven strategy to boost application performance, and Redis serves as an excellent caching solution.
By storing frequently accessed data in this database, full-stack developers can reduce database load and minimize response times, leading to a smoother user experience.
// Example of using Redis for caching user data
const userId = '123';
const cacheKey = `user:${userId}`;
// Check if user data is in the cache
client.get(cacheKey, (err, cachedData) => {
if (cachedData) {
// Use cached data
console.log('User data retrieved from cache:', JSON.parse(cachedData));
} else {
// Fetch user data from the database and store it in Redis for future use
const userData = fetchUserDataFromDatabase(userId);
client.set(cacheKey, JSON.stringify(userData));
console.log('User data retrieved from the database:', userData);
}
});
Strategies for Integrating Redis Caching in Full-Stack Environment
Imagine an e-commerce website displaying product listings. By caching product data in this database, the application can serve frequently requested information quickly, reducing the load on the database and ensuring a responsive user interface.
Its seamless integration with caching strategies makes it an invaluable tool for full-stack developers aiming to optimize their applications.
As we approach the conclusion, we’ll delve into the strategic implementation of It and guide aspiring full-stack web developers on when to consider integrating this powerful tool into their projects.
#6 – Redis and Microservices
Redis Complementing Microservices Architecture
Microservices have become a prevalent architectural pattern in modern full-stack web development.
Seamlessly complements microservices by providing a reliable and efficient means of communication between different service components.
Its Pub/Sub mechanism, combined with in-memory storage, enables microservices to communicate in real-time.
// Example of using Redis for inter-microservice communication
const channel = 'microservice:events';
// Service A publishes an event
client.publish(channel, 'Event from Service A: Data updated');
// Service B subscribes to the channel and reacts to the event
client.subscribe(channel);
client.on('message', (channel, message) => {
console.log(`Received event on channel ${channel}: ${message}`);
});
Examples of Microservices Benefiting from Redis Integration
Consider a scenario where multiple microservices collaborate to process user orders in an e-commerce platform.
Facilitates seamless communication between these microservices, ensuring that order updates and status changes are broadcasted in real-time.
This integration enhances the overall responsiveness and reliability of the microservices architecture.
In the final section, we’ll explore the strategic aspects of implementing Redis in full-stack projects.
#7 – Strategic Implementation
When to Consider Integrating Redis in a Full-Stack Project
Choosing the right tools for a full-stack project is a crucial decision. Redis becomes particularly advantageous in scenarios where speed, real-time communication, and scalability are paramount.
Consider integrating Redis when your application demands:
- Rapid Data Retrieval: If your application requires quick access to frequently accessed data, its in-memory storage provides a significant performance boost.
- Real-time Features: For applications where real-time updates and interactivity are crucial, such as chat applications or collaborative editing platforms, its Pub/Sub mechanism proves invaluable.
- Scalability Needs: As your user base grows, its ability to handle large datasets and distribute workloads across multiple instances ensures seamless scalability.
Factors to Evaluate Before Choosing Redis
While it offers numerous advantages, it’s essential to assess whether it aligns with your project’s specific requirements. Consider the following factors:
- Data Persistence: It is primarily operating in-memory, so evaluate if data persistence is a critical requirement for your application. It provides options for persistence, but it’s essential to weigh the trade-offs.
- Complex Querying Needs: If your application heavily relies on complex queries and relational data, a traditional relational database might be more suitable.
- Resource Utilization: Assess the resources available in your hosting environment, as its in-memory nature may demand additional server capacity.
Aspiring full-stack web developers, by strategically considering these factors, you can make informed decisions on when to integrate it into your projects for optimal outcomes.
Conclusion
In full-stack web development, the strategic choices we make in our technology stack can profoundly impact the performance and user experience of our applications.
With its impressive speed, data structure flexibility, scalability, and real-time capabilities, emerges as a compelling addition to the toolkit of aspiring full-stack developers.
Whether you’re building a social media platform, an e-commerce website, or any other dynamic application, Redis provides the tools to elevate your project to new heights.
Consider Redis not just as a database choice but as a strategic partner in crafting high-performance, responsive, and scalable applications.
Experiment with the example codes provided, explore Redis documentation, and witness firsthand the advantages it brings to your projects.
Happy Coding!
Leave a Reply